[GitHub] [incubator-iotdb] liutaohua commented on a change in pull request #1650: [IOTDB-848] support order by time asc/desc
liutaohua commented on a change in pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#discussion_r486789846 ## File path: server/src/main/java/org/apache/iotdb/db/query/dataset/groupby/GroupByWithValueFilterDataSet.java ## @@ -122,13 +127,18 @@ protected RowRecord nextWithoutConstraint() throws IOException { long[] timestampArray = new long[timeStampFetchSize]; int timeArrayLength = 0; -if (hasCachedTimestamp) { +while (!cachedTimestamps.isEmpty()) { + long timestamp = cachedTimestamps.remove(); if (timestamp < curEndTime) { +if (!groupByTimePlan.isAscending() && timestamp < curStartTime) { + cachedTimestamps.addFirst(timestamp); + return constructRowRecord(aggregateResultList); +} if (timestamp >= curStartTime) { - hasCachedTimestamp = false; timestampArray[timeArrayLength++] = timestamp; } } else { +cachedTimestamps.addFirst(timestamp); Review comment: i think we need put it back , for example , the interval is [0,10) , [10,20), [20,30), if the first cache is 30 ,and the end time is 10, so that need put back This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] HTHou commented on pull request #1524: [IOTDB-776] Control the memory usage of flushing the memtable
HTHou commented on pull request #1524: URL: https://github.com/apache/incubator-iotdb/pull/1524#issuecomment-690877249 > please add the design doc. > @HTHou I'm working on it. It'll be available to share once it's finished. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] HTHou merged pull request #1702: fix mem setting on windows when using 64-bit Java
HTHou merged pull request #1702: URL: https://github.com/apache/incubator-iotdb/pull/1702 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486764762 ## File path: server/src/main/java/org/apache/iotdb/db/metadata/logfile/MLogWriter.java ## @@ -0,0 +1,350 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one + * or more contributor license agreements. See the NOTICE file + * distributed with this work for additional information + * regarding copyright ownership. The ASF licenses this file + * to you under the Apache License, Version 2.0 (the + * "License"); you may not use this file except in compliance + * with the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, + * software distributed under the License is distributed on an + * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY + * KIND, either express or implied. See the License for the + * specific language governing permissions and limitations + * under the License. + */ +package org.apache.iotdb.db.metadata.logfile; + +import java.io.*; +import java.nio.BufferOverflowException; +import java.nio.ByteBuffer; +import java.nio.file.Files; +import java.util.*; + +import org.apache.iotdb.db.conf.IoTDBDescriptor; +import org.apache.iotdb.db.engine.fileSystem.SystemFileFactory; +import org.apache.iotdb.db.exception.metadata.MetadataException; +import org.apache.iotdb.db.metadata.MetadataConstant; +import org.apache.iotdb.db.metadata.MetadataOperationType; +import org.apache.iotdb.db.metadata.PartialPath; +import org.apache.iotdb.db.metadata.mnode.MNode; +import org.apache.iotdb.db.metadata.mnode.MeasurementMNode; +import org.apache.iotdb.db.metadata.mnode.StorageGroupMNode; +import org.apache.iotdb.db.qp.physical.PhysicalPlan; +import org.apache.iotdb.db.qp.physical.sys.*; +import org.apache.iotdb.db.writelog.io.LogWriter; +import org.apache.iotdb.tsfile.file.metadata.enums.CompressionType; +import org.apache.iotdb.tsfile.file.metadata.enums.TSDataType; +import org.apache.iotdb.tsfile.file.metadata.enums.TSEncoding; +import org.apache.iotdb.tsfile.fileSystem.FSFactoryProducer; +import org.slf4j.Logger; +import org.slf4j.LoggerFactory; + +public class MLogWriter { + + private static final Logger logger = LoggerFactory.getLogger(MLogWriter.class); + private File logFile; + private LogWriter logWriter; + private int logNum; + private ByteBuffer mlogBuffer = ByteBuffer.allocate( +IoTDBDescriptor.getInstance().getConfig().getMlogBufferSize()); + + public MLogWriter(String schemaDir, String logFileName) throws IOException { +File metadataDir = SystemFileFactory.INSTANCE.getFile(schemaDir); +if (!metadataDir.exists()) { + if (metadataDir.mkdirs()) { +logger.info("create schema folder {}.", metadataDir); + } else { +logger.info("create schema folder {} failed.", metadataDir); + } +} + +logFile = SystemFileFactory.INSTANCE.getFile(schemaDir + File.separator + logFileName); +// always flush +logWriter = new LogWriter(logFile, 0L); + } + + public MLogWriter(String logFilePath) throws IOException { +logFile = SystemFileFactory.INSTANCE.getFile(logFilePath); +// always flush +logWriter = new LogWriter(logFile, 0L); + } + + public void close() throws IOException { +sync(); +logWriter.close(); + } + + private void sync() { +try { + logWriter.write(mlogBuffer); +} catch (IOException e) { + logger.error("MLog {} sync failed, change system mode to read-only", logFile.getAbsoluteFile(), e); + IoTDBDescriptor.getInstance().getConfig().setReadOnly(true); +} +mlogBuffer.clear(); + } + + private void putLog(PhysicalPlan plan) { +mlogBuffer.mark(); +try { + plan.serialize(mlogBuffer); +} catch (BufferOverflowException e) { + logger.error("MLog {} BufferOverflow !", plan.getOperatorType(), e); + mlogBuffer.reset(); + sync(); + plan.serialize(mlogBuffer); +} +logNum ++; + } + + public void createTimeseries(CreateTimeSeriesPlan plan, long offset) throws IOException { +try { + putLog(plan); + ChangeTagOffsetPlan changeTagOffsetPlan = new ChangeTagOffsetPlan(plan.getPath(), offset); + putLog(changeTagOffsetPlan); +} catch (BufferOverflowException e) { + throw new IOException( +"Log cannot fit into buffer, please increase mlog_buffer_size", e); +} + } + + public void deleteTimeseries(DeleteTimeSeriesPlan deleteTimeSeriesPlan) throws IOException { +try { + putLog(deleteTimeSeriesPlan); +} catch (BufferOverflowException e) { + throw new IOException( +"Log cannot fit into buffer, please increase mlog_buffer_size", e); +} + } + + public void setStorageGroup(PartialPath storageGroup) throws IOException { +try { + SetStorageGroupPlan plan = new SetStorageGroupPlan(storageGroup); +
[GitHub] [incubator-iotdb] haimeiguo opened a new pull request #1722: [iotdb-872] Use system timezone in CLI
haimeiguo opened a new pull request #1722: URL: https://github.com/apache/incubator-iotdb/pull/1722 cli timezone will be set to cli local timezone by default, instead of server timezone This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] removed a comment on pull request #1524: [IOTDB-776] Control the memory usage of flushing the memtable
sonarcloud[bot] removed a comment on pull request #1524: URL: https://github.com/apache/incubator-iotdb/pull/1524#issuecomment-687038561 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1524=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=CODE_SMELL) [10 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1524) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1524=new_duplicated_lines_density=list) [0.4% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1524=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] commented on pull request #1524: [IOTDB-776] Control the memory usage of flushing the memtable
sonarcloud[bot] commented on pull request #1524: URL: https://github.com/apache/incubator-iotdb/pull/1524#issuecomment-690860711 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1524=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=CODE_SMELL) [10 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1524=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1524) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1524=new_duplicated_lines_density=list) [0.4% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1524=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] removed a comment on pull request #1650: [IOTDB-848] support order by time asc/desc
sonarcloud[bot] removed a comment on pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#issuecomment-690034269 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [2 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) [0.0% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] commented on pull request #1650: [IOTDB-848] support order by time asc/desc
sonarcloud[bot] commented on pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#issuecomment-690857074 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [2 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) [0.0% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] Genius-pig commented on pull request #1717: [IOTDB-881] Fix Double quotes bug in Align by device
Genius-pig commented on pull request #1717: URL: https://github.com/apache/incubator-iotdb/pull/1717#issuecomment-690856050 Can you add support nested double quotes in `Path`? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] JackieTien97 commented on a change in pull request #1650: [IOTDB-848] support order by time asc/desc
JackieTien97 commented on a change in pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#discussion_r486749377 ## File path: server/src/main/java/org/apache/iotdb/db/query/dataset/groupby/GroupByWithValueFilterDataSet.java ## @@ -122,13 +127,18 @@ protected RowRecord nextWithoutConstraint() throws IOException { long[] timestampArray = new long[timeStampFetchSize]; int timeArrayLength = 0; -if (hasCachedTimestamp) { +while (!cachedTimestamps.isEmpty()) { + long timestamp = cachedTimestamps.remove(); if (timestamp < curEndTime) { +if (!groupByTimePlan.isAscending() && timestamp < curStartTime) { + cachedTimestamps.addFirst(timestamp); + return constructRowRecord(aggregateResultList); +} if (timestamp >= curStartTime) { - hasCachedTimestamp = false; timestampArray[timeArrayLength++] = timestamp; } } else { +cachedTimestamps.addFirst(timestamp); Review comment: It seems that this timestamp is useless, we don't need to put it back to `cachedTimestamps`. ## File path: server/src/main/java/org/apache/iotdb/db/query/dataset/groupby/GroupByWithValueFilterDataSet.java ## @@ -122,13 +127,18 @@ protected RowRecord nextWithoutConstraint() throws IOException { long[] timestampArray = new long[timeStampFetchSize]; int timeArrayLength = 0; -if (hasCachedTimestamp) { +while (!cachedTimestamps.isEmpty()) { + long timestamp = cachedTimestamps.remove(); if (timestamp < curEndTime) { +if (!groupByTimePlan.isAscending() && timestamp < curStartTime) { + cachedTimestamps.addFirst(timestamp); + return constructRowRecord(aggregateResultList); +} if (timestamp >= curStartTime) { - hasCachedTimestamp = false; timestampArray[timeArrayLength++] = timestamp; Review comment: It seems there will be IndexOutOfArrayException here. What if `cachedTimestamps`'s size is larger than `timeStampFetchSize`. And also, you call the `constructTimeArrayForOneCal` method in the following and fetch another `timeStampFetchSize` timestamp, i think it should be changed in case of `desc`. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] jt2594838 commented on a change in pull request #1691: [IOTDB-814] Cache and redirect distributed IoTDB's leader for write requests in IoTDB's client
jt2594838 commented on a change in pull request #1691: URL: https://github.com/apache/incubator-iotdb/pull/1691#discussion_r486728689 ## File path: cluster/src/main/java/org/apache/iotdb/cluster/server/member/MetaGroupMember.java ## @@ -1688,13 +1693,20 @@ private TSStatus forwardInsertTabletPlan(Map planG TSStatus[] subStatus = null; boolean noFailure = true; boolean isBatchFailure = false; +boolean allRedirect = true; +EndPoint endPoint = null; for (Map.Entry entry : planGroupMap.entrySet()) { tmpStatus = forwardToSingleGroup(entry); logger.debug("{}: from {},{},{}", name, entry.getKey(), entry.getValue(), tmpStatus); noFailure = (tmpStatus.getCode() == TSStatusCode.SUCCESS_STATUS.getStatusCode()) && noFailure; isBatchFailure = (tmpStatus.getCode() == TSStatusCode.MULTIPLE_ERROR.getStatusCode()) || isBatchFailure; + if (tmpStatus.isSetRedirectNode()) { +endPoint = tmpStatus.getRedirectNode(); + } else { +allRedirect = false; + } Review comment: I think the redirect strategy can be improved a little. Mostly the plan is forwarded to multiple groups because its data has crossed multiple time partitions, and generally, this only happens on time partition borders as a plan contains a relatively short time range compared to the time partition length. So typically `planGroupMap.size() <= 2`, and when it is 2, the following plans most probably belong to the second group. Consequently, it would reasonable if we always set the redirect target to that of the sub-plan with the largest timestamp. For example, if the partition length is a day, and a plan that contains data from Thuesday 11:50 to Wednesday 00:10 will be split into two sub-plans, each contains data from Thuesday 11:50 to Thuesday 11:59 and Wednesday 00:00 to Wednesday 00:10 respectively, and the two sub-plans are forwarded to NodeA and NodeB. It is clear that the following plans, which contain data of Wednesday, should be forwarded to NodeB. By the way, I think the use of a map to split plan is unnecessary, and a `List>` should be just enough, and you may sort it according to the largest timestamp easily. ## File path: session/src/main/java/org/apache/iotdb/session/Session.java ## @@ -121,209 +105,326 @@ private synchronized void open(boolean enableRPCCompression, int connectionTimeo if (!isClosed) { return; } +this.enableRPCCompression = enableRPCCompression; +this.connectionTimeoutInMs = connectionTimeoutInMs; -transport = new TFastFramedTransport(new TSocket(host, port, connectionTimeoutInMs)); +defaultSessionConnection = new SessionConnection(this, defaultEndPoint); +metaSessionConnection = defaultSessionConnection; +isClosed = false; +if (Config.DEFAULT_CACHE_LEADER_MODE) { + deviceIdToEndpoint = new HashMap<>(); + endPointToSessionConnection = new HashMap() {{ +put(defaultEndPoint, defaultSessionConnection); + }}; +} + } -if (!transport.isOpen()) { - try { -transport.open(); - } catch (TTransportException e) { -throw new IoTDBConnectionException(e); + public synchronized void close() throws IoTDBConnectionException { +if (isClosed) { + return; +} +try { + if (Config.DEFAULT_CACHE_LEADER_MODE) { +for (SessionConnection sessionConnection : endPointToSessionConnection.values()) { + sessionConnection.close(); +} + } else { +defaultSessionConnection.close(); } +} finally { + isClosed = true; } + } -if (enableRPCCompression) { - client = new TSIService.Client(new TCompactProtocol(transport)); -} else { - client = new TSIService.Client(new TBinaryProtocol(transport)); -} + public synchronized String getTimeZone() + throws StatementExecutionException, IoTDBConnectionException { +return defaultSessionConnection.getTimeZone(); + } -TSOpenSessionReq openReq = new TSOpenSessionReq(); -openReq.setUsername(username); -openReq.setPassword(password); + public synchronized void setTimeZone(String zoneId) + throws StatementExecutionException, IoTDBConnectionException { +defaultSessionConnection.setTimeZone(zoneId); + } + public void setStorageGroup(String storageGroup) + throws IoTDBConnectionException, StatementExecutionException { try { - TSOpenSessionResp openResp = client.openSession(openReq); + metaSessionConnection.setStorageGroup(storageGroup); +} catch (RedirectException e) { + if (Config.DEFAULT_CACHE_LEADER_MODE) { +logger.debug("storageGroup[{}]:{}", storageGroup, e.getMessage()); +metaSessionConnection = new SessionConnection(this, e.getEndPoint()); +endPointToSessionConnection.putIfAbsent(e.getEndPoint(), metaSessionConnection); + } +} + }
[GitHub] [incubator-iotdb] qiaojialin commented on a change in pull request #1650: [IOTDB-848] support order by time asc/desc
qiaojialin commented on a change in pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#discussion_r486748299 ## File path: docs/UserGuide/Operation Manual/DML Data Manipulation Language.md ## @@ -146,6 +146,15 @@ The selected timeseries are "the power supply status of ln group wf01 plant wt01 The execution result of this SQL statement is as follows: https://user-images.githubusercontent.com/13203019/51577450-dcfe0800-1ef4-11e9-9399-4ba2b2b7fb73.jpg;> + + Order By Time Query +IoTDB supports the 'order by time' statement after 0.10.x, it's used to display results in descending order by time. Review comment: ```suggestion IoTDB supports the 'order by time' statement since 0.11, it's used to display results in descending order by time. ``` This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] yhwang-hbl commented on a change in pull request #1710: Supplement the document
yhwang-hbl commented on a change in pull request #1710: URL: https://github.com/apache/incubator-iotdb/pull/1710#discussion_r486747584 ## File path: docs/zh/UserGuide/Operation Manual/DDL Data Definition Language.md ## @@ -41,14 +41,15 @@ Msg: org.apache.iotdb.exception.MetadataErrorException: org.apache.iotdb.excepti ## 查看存储组 -在存储组创建后,我们可以使用[SHOW STORAGE GROUP](../Operation%20Manual/SQL%20Reference.md)语句来查看所有的存储组,SQL语句如下所示: +在存储组创建后,我们可以使用[SHOW STORAGE GROUP](../Operation%20Manual/SQL%20Reference.md)语句和[SHOW STORAGE GROUP ](../Operation%20Manual/SQL%20Reference.md)来查看存储组,SQL语句如下所示: Review comment: Copy that ,i will improve it This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] yhwang-hbl commented on a change in pull request #1710: Supplement the document
yhwang-hbl commented on a change in pull request #1710: URL: https://github.com/apache/incubator-iotdb/pull/1710#discussion_r486747363 ## File path: docs/UserGuide/Operation Manual/DDL Data Definition Language.md ## @@ -309,6 +310,20 @@ IoTDB> unset ttl to root.ln After unset TTL, all data will be accepted in `root.ln` +## Show TTL Review comment: Hi, I changed the Chinese document in the PR submitted earlier, This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] yhwang-hbl commented on a change in pull request #1710: Supplement the document
yhwang-hbl commented on a change in pull request #1710: URL: https://github.com/apache/incubator-iotdb/pull/1710#discussion_r486747467 ## File path: docs/UserGuide/Operation Manual/DDL Data Definition Language.md ## @@ -309,6 +310,20 @@ IoTDB> unset ttl to root.ln After unset TTL, all data will be accepted in `root.ln` +## Show TTL Review comment: > same as neuyilan's suggestion~ Hi, I changed the Chinese document in the PR submitted earlier, This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486742681 ## File path: server/src/main/java/org/apache/iotdb/db/metadata/MTree.java ## @@ -1002,55 +1008,74 @@ private void findNodes(MNode node, PartialPath path, List res, int } public void serializeTo(String snapshotPath) throws IOException { -try (BufferedWriter bw = new BufferedWriter( -new FileWriter(SystemFileFactory.INSTANCE.getFile(snapshotPath { - root.serializeTo(bw); +MLogWriter mLogWriter = null; +try { + mLogWriter = new MLogWriter(snapshotPath); + root.serializeTo(mLogWriter); +} finally { + if (mLogWriter != null) { +mLogWriter.close(); + } } } @SuppressWarnings("squid:S3776") // Suppress high Cognitive Complexity warning public static MTree deserializeFrom(File mtreeSnapshot) { -try (BufferedReader br = new BufferedReader(new FileReader(mtreeSnapshot))) { - String s; +MLogReader mlogReader = null; +try { Review comment: Got it! This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486742648 ## File path: server/src/main/java/org/apache/iotdb/db/conf/IoTDBConfigCheck.java ## @@ -203,6 +203,13 @@ public void checkConfig() throws IOException { } // rename tmpLogFile to mlog FileUtils.moveFile(tmpMLogFile, mlogFile); + + File oldMLogFile = SystemFileFactory.INSTANCE.getFile(SCHEMA_DIR + File.separator + + MetadataConstant.METADATA_OLD_LOG); Review comment: yes, it's no meaning. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] LebronAl edited a comment on pull request #1691: [IOTDB-814] Cache and redirect distributed IoTDB's leader for write requests in IoTDB's client
LebronAl edited a comment on pull request #1691: URL: https://github.com/apache/incubator-iotdb/pull/1691#issuecomment-689985530 # Benchmark Parameter CLIENT_NUMBER=1 GROUP_NUMBER=1 DEVICE_NUMBER=20 SENSOR_NUMBER=20 BATCH_SIZE=50 LOOP=1000 DATA_TYPE=DOUBLE INSERT_MODE=session # Cluster config default_replica_num=1 enablePartition=false is_enable_raft_log_persistence=true is_use_async_applier=true two nodes's cluster(34,35) # Cluster_new's latest Version(df8e0891cb75e0c6ff61ce10aedbaa8b99b66a31 add documents) ## forward(benchmark writes data to 34, sg belongs to 35,internal forward) 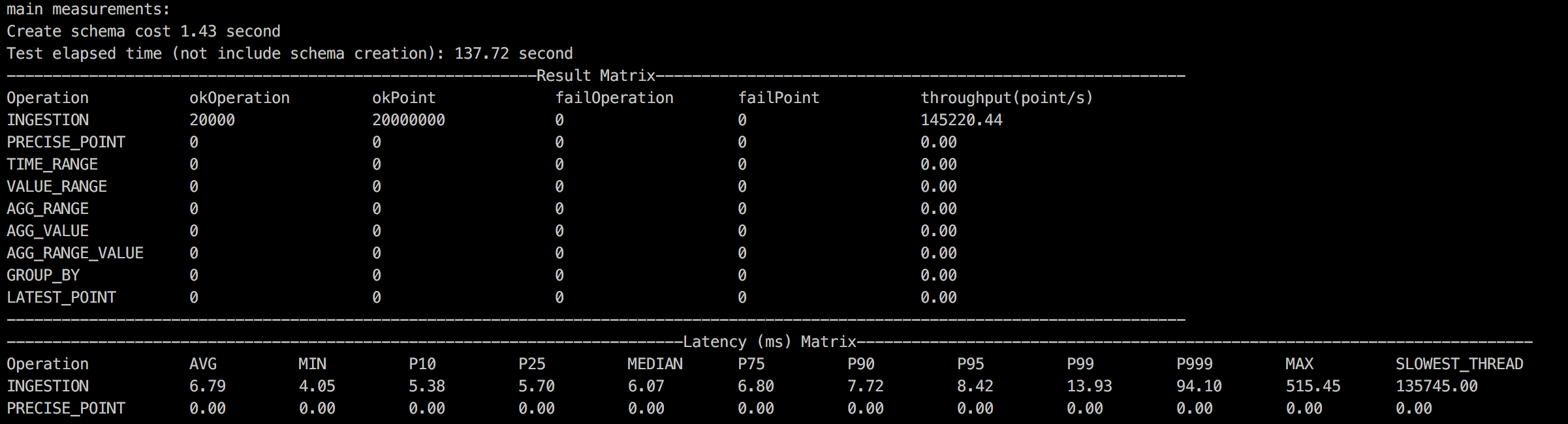 ## non-forward(benchmark writes data to 35, sg belongs to 35,no need to forward) 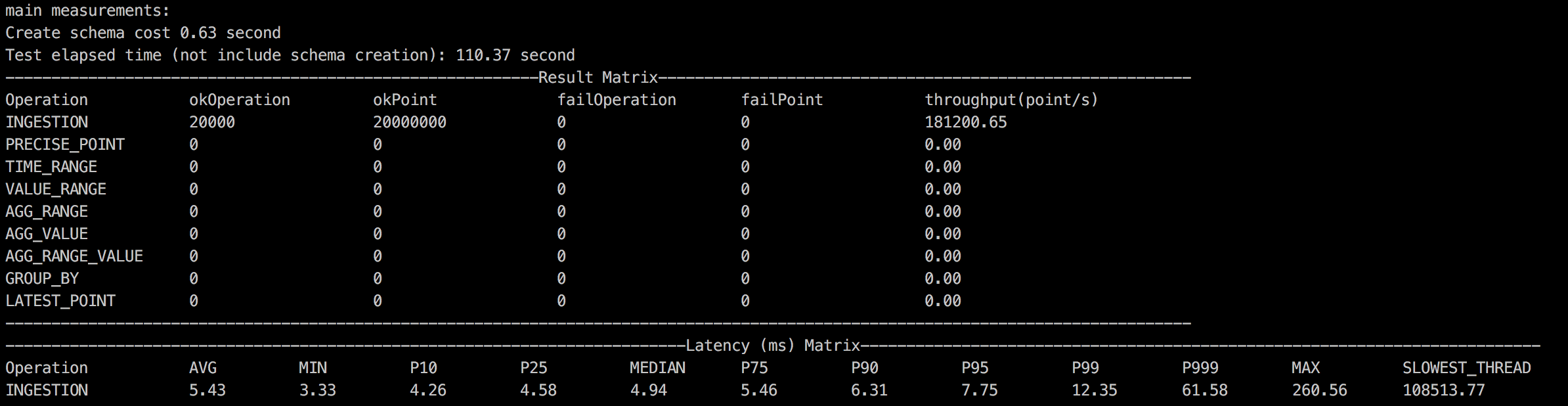 # Cluster_new_cache_leader's latest Version ## Close cache leader ### forward(benchmark writes data to 34, sg belongs to 35,internal forward) 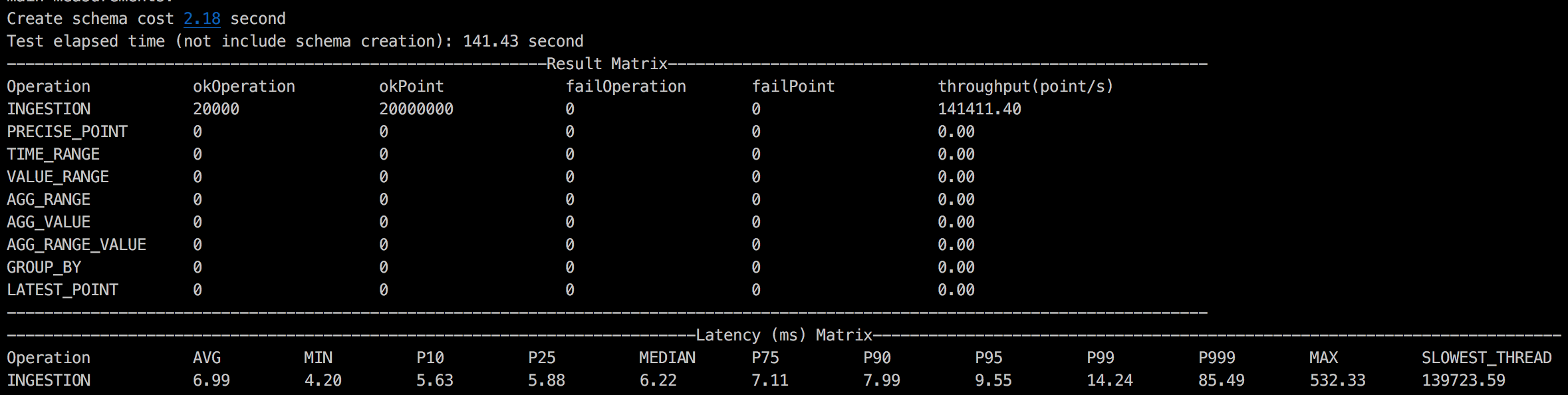 ### non-forward(benchmark writes data to 35, sg belongs to 35,no need to forward) 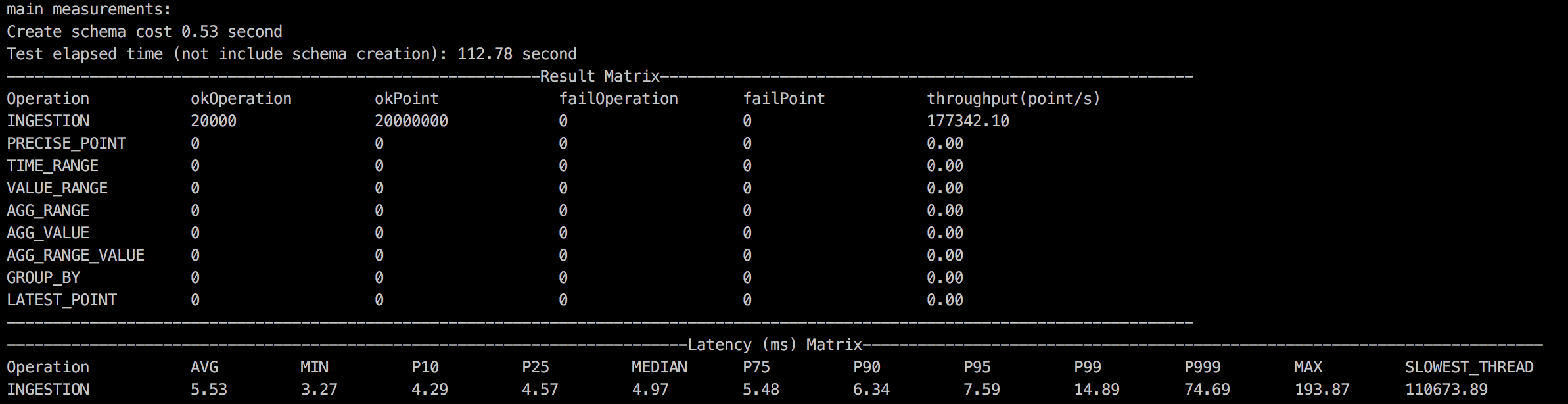 ## Open cache leader ### little forward(benchmark writes data to 34, sg belongs to 35,internal forward first write for each deviceId + cache leader) 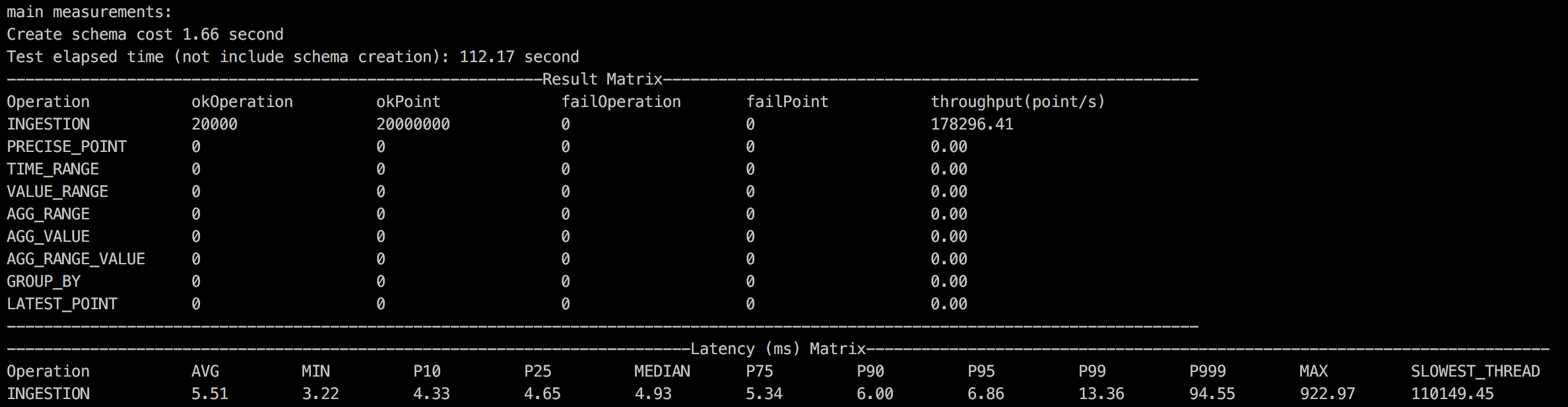 ### non-forward(benchmark writes data to 35, sg belongs to 35,no need to forward) 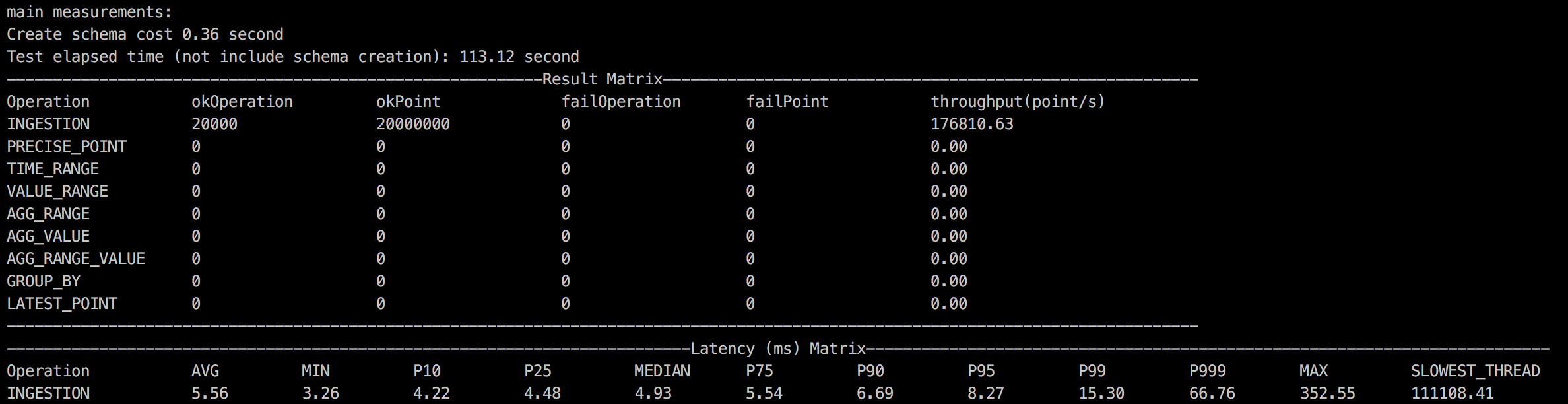 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486740857 ## File path: docs/zh/SystemDesign/SchemaManager/SchemaManager.md ## @@ -211,7 +211,7 @@ IoTDB 的元数据管理采用目录树的形式,倒数第二层为设备层 ## 元数据日志管理 -* org.apache.iotdb.db.metadata.MLogWriter +* org.apache.iotdb.db.metadata.logfile.MLogWriter 所有元数据的操作均会记录到元数据日志文件中,此文件默认为 data/system/schema/mlog.txt。 Review comment: Got it! This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] HTHou commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
HTHou commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486726741 ## File path: server/src/main/java/org/apache/iotdb/db/conf/IoTDBConfigCheck.java ## @@ -203,6 +203,13 @@ public void checkConfig() throws IOException { } // rename tmpLogFile to mlog FileUtils.moveFile(tmpMLogFile, mlogFile); + + File oldMLogFile = SystemFileFactory.INSTANCE.getFile(SCHEMA_DIR + File.separator + + MetadataConstant.METADATA_OLD_LOG); + + if (oldMLogFile.delete()) { +throw new IOException("Deleting old mlog " + oldMLogFile + "failed."); + } Review comment: Should be `!oldMLogFile.delete()` here? ## File path: docs/SystemDesign/SchemaManager/SchemaManager.md ## @@ -213,7 +213,7 @@ The method is `MManager.initFromLog()`: ## Log management of metadata -* org.apache.iotdb.db.metadata.MLogWriter +* org.apache.iotdb.db.metadata.logfile.MLogWriter All metadata operations are recorded in a metadata log file, which defaults to data/system/schema/mlog.txt. Review comment: ```suggestion All metadata operations are recorded in a metadata log file, which defaults to data/system/schema/mlog.bin. ``` ## File path: server/src/main/java/org/apache/iotdb/db/metadata/MTree.java ## @@ -1002,55 +1008,74 @@ private void findNodes(MNode node, PartialPath path, List res, int } public void serializeTo(String snapshotPath) throws IOException { -try (BufferedWriter bw = new BufferedWriter( -new FileWriter(SystemFileFactory.INSTANCE.getFile(snapshotPath { - root.serializeTo(bw); +MLogWriter mLogWriter = null; +try { + mLogWriter = new MLogWriter(snapshotPath); + root.serializeTo(mLogWriter); +} finally { + if (mLogWriter != null) { +mLogWriter.close(); + } } Review comment: Use try with resource ## File path: server/src/main/java/org/apache/iotdb/db/metadata/MTree.java ## @@ -1002,55 +1008,74 @@ private void findNodes(MNode node, PartialPath path, List res, int } public void serializeTo(String snapshotPath) throws IOException { -try (BufferedWriter bw = new BufferedWriter( -new FileWriter(SystemFileFactory.INSTANCE.getFile(snapshotPath { - root.serializeTo(bw); +MLogWriter mLogWriter = null; +try { + mLogWriter = new MLogWriter(snapshotPath); + root.serializeTo(mLogWriter); +} finally { + if (mLogWriter != null) { +mLogWriter.close(); + } } } @SuppressWarnings("squid:S3776") // Suppress high Cognitive Complexity warning public static MTree deserializeFrom(File mtreeSnapshot) { -try (BufferedReader br = new BufferedReader(new FileReader(mtreeSnapshot))) { - String s; +MLogReader mlogReader = null; +try { Review comment: Use try with resource to avoid new sonar code smell.. ## File path: docs/zh/SystemDesign/SchemaManager/SchemaManager.md ## @@ -211,7 +211,7 @@ IoTDB 的元数据管理采用目录树的形式,倒数第二层为设备层 ## 元数据日志管理 -* org.apache.iotdb.db.metadata.MLogWriter +* org.apache.iotdb.db.metadata.logfile.MLogWriter 所有元数据的操作均会记录到元数据日志文件中,此文件默认为 data/system/schema/mlog.txt。 Review comment: ```suggestion 所有元数据的操作均会记录到元数据日志文件中,此文件默认为 data/system/schema/mlog.bin。 ``` ## File path: server/src/main/java/org/apache/iotdb/db/metadata/MTree.java ## @@ -1002,55 +1008,74 @@ private void findNodes(MNode node, PartialPath path, List res, int } public void serializeTo(String snapshotPath) throws IOException { -try (BufferedWriter bw = new BufferedWriter( -new FileWriter(SystemFileFactory.INSTANCE.getFile(snapshotPath { - root.serializeTo(bw); +MLogWriter mLogWriter = null; +try { + mLogWriter = new MLogWriter(snapshotPath); + root.serializeTo(mLogWriter); +} finally { + if (mLogWriter != null) { +mLogWriter.close(); + } } } @SuppressWarnings("squid:S3776") // Suppress high Cognitive Complexity warning public static MTree deserializeFrom(File mtreeSnapshot) { -try (BufferedReader br = new BufferedReader(new FileReader(mtreeSnapshot))) { - String s; +MLogReader mlogReader = null; +try { + mlogReader = new MLogReader(mtreeSnapshot); Deque nodeStack = new ArrayDeque<>(); MNode node = null; - while ((s = br.readLine()) != null) { -String[] nodeInfo = s.split(","); -short nodeType = Short.parseShort(nodeInfo[0]); -if (nodeType == MetadataConstant.STORAGE_GROUP_MNODE_TYPE) { - node = StorageGroupMNode.deserializeFrom(nodeInfo); -} else if (nodeType == MetadataConstant.MEASUREMENT_MNODE_TYPE) { - node = MeasurementMNode.deserializeFrom(nodeInfo); -} else { - node = new MNode(null, nodeInfo[1]); -} + while
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486737390 ## File path: server/src/main/java/org/apache/iotdb/db/qp/physical/sys/StorageGroupMNodePlan.java ## @@ -0,0 +1,124 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one + * or more contributor license agreements. See the NOTICE file + * distributed with this work for additional information + * regarding copyright ownership. The ASF licenses this file + * to you under the Apache License, Version 2.0 (the + * "License"); you may not use this file except in compliance + * with the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, + * software distributed under the License is distributed on an + * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY + * KIND, either express or implied. See the License for the + * specific language governing permissions and limitations + * under the License. + */ + +package org.apache.iotdb.db.qp.physical.sys; + +import org.apache.iotdb.db.metadata.PartialPath; +import org.apache.iotdb.db.qp.logical.Operator; +import org.apache.iotdb.db.qp.physical.PhysicalPlan; + +import java.io.DataOutputStream; +import java.io.IOException; +import java.nio.ByteBuffer; +import java.util.ArrayList; +import java.util.List; +import java.util.Objects; + +public class StorageGroupMNodePlan extends PhysicalPlan { Review comment: haha, I will change it. It's a mistake. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486737016 ## File path: server/src/test/java/org/apache/iotdb/db/integration/IoTDBCreateSnapshotIT.java ## @@ -156,4 +178,20 @@ private void checkShowTimeseries(Statement statement) throws SQLException { Assert.assertEquals(8, cnt); } } + + private PhysicalPlan convertFromString(String str) { +String[] words = str.split(","); +if (words[0].equals("2")) { Review comment: ok, thanks! ## File path: server/src/main/java/org/apache/iotdb/db/sync/receiver/transfer/SyncServiceImpl.java ## @@ -272,18 +274,25 @@ public SyncStatus checkDataMD5(String md5OfSender) throws TException { private void loadMetadata() { logger.info("Start to load metadata in sync process."); if (currentFile.get().exists()) { - try (BufferedReader br = new BufferedReader( - new java.io.FileReader(currentFile.get( { -String metadataOperation; -while ((metadataOperation = br.readLine()) != null) { + MLogReader mLogReader = null; + try { +mLogReader = new MLogReader(config.getSchemaDir(), MetadataConstant.METADATA_LOG); +while (mLogReader.hasNext()) { + PhysicalPlan plan = mLogReader.next(); try { -IoTDB.metaManager.operation(metadataOperation); - } catch (IOException | MetadataException e) { -logger.error("Can not operate metadata operation {} ", metadataOperation, e); +if (plan == null) { + continue; +} +IoTDB.metaManager.operation(plan); + } catch (Exception e) { +logger.error("Can not operate metadata operation {} for err:{}", plan.getOperatorType(), e); } } +mLogReader.close(); } catch (IOException e) { logger.error("Cannot read the file {}.", currentFile.get().getAbsoluteFile(), e); + } finally { +mLogReader.close(); Review comment: yes! This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486736939 ## File path: server/src/main/java/org/apache/iotdb/db/writelog/io/LogWriter.java ## @@ -41,13 +42,26 @@ private IoTDBConfig config = IoTDBDescriptor.getInstance().getConfig(); private ByteBuffer lengthBuffer = ByteBuffer.allocate(4); private ByteBuffer checkSumBuffer = ByteBuffer.allocate(8); + private long forcePeriodInMs = 0; - public LogWriter(String logFilePath) { + public LogWriter(String logFilePath, long forcePeriodInMs) throws FileNotFoundException { logFile = SystemFileFactory.INSTANCE.getFile(logFilePath); +this.forcePeriodInMs = forcePeriodInMs; + +if (channel == null) { + fileOutputStream = new FileOutputStream(logFile, true); + channel = fileOutputStream.getChannel(); +} } - public LogWriter(File logFile) { + public LogWriter(File logFile, long forcePeriodInMs) throws FileNotFoundException { this.logFile = logFile; +this.forcePeriodInMs = forcePeriodInMs; + +if (channel == null) { + fileOutputStream = new FileOutputStream(logFile, true); Review comment: I think we should throw the exception for it's not normal, because fileoutputstream could create it if not exist. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486736400 ## File path: server/src/main/java/org/apache/iotdb/db/conf/IoTDBConfigCheck.java ## @@ -203,6 +203,13 @@ public void checkConfig() throws IOException { } // rename tmpLogFile to mlog FileUtils.moveFile(tmpMLogFile, mlogFile); + + File oldMLogFile = SystemFileFactory.INSTANCE.getFile(SCHEMA_DIR + File.separator + + MetadataConstant.METADATA_OLD_LOG); + + if (oldMLogFile.delete()) { Review comment: yes, thank you for pointing this out. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486736109 ## File path: server/src/main/java/org/apache/iotdb/db/writelog/io/LogWriter.java ## @@ -41,13 +42,26 @@ private IoTDBConfig config = IoTDBDescriptor.getInstance().getConfig(); private ByteBuffer lengthBuffer = ByteBuffer.allocate(4); private ByteBuffer checkSumBuffer = ByteBuffer.allocate(8); + private long forcePeriodInMs = 0; - public LogWriter(String logFilePath) { + public LogWriter(String logFilePath, long forcePeriodInMs) throws FileNotFoundException { logFile = SystemFileFactory.INSTANCE.getFile(logFilePath); +this.forcePeriodInMs = forcePeriodInMs; + +if (channel == null) { + fileOutputStream = new FileOutputStream(logFile, true); + channel = fileOutputStream.getChannel(); +} } Review comment: Yes, I will add some comments. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] liukun4515 commented on pull request #1524: [IOTDB-776] Control the memory usage of flushing the memtable
liukun4515 commented on pull request #1524: URL: https://github.com/apache/incubator-iotdb/pull/1524#issuecomment-690829483 please add the design doc. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] liukun4515 edited a comment on pull request #1524: [IOTDB-776] Control the memory usage of flushing the memtable
liukun4515 edited a comment on pull request #1524: URL: https://github.com/apache/incubator-iotdb/pull/1524#issuecomment-690829483 please add the design doc. @HTHou This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] samperson1997 commented on a change in pull request #1721: [IOTDB-868] fix comma bug for mlog
samperson1997 commented on a change in pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721#discussion_r486721209 ## File path: server/src/main/java/org/apache/iotdb/db/conf/IoTDBConfigCheck.java ## @@ -203,6 +203,13 @@ public void checkConfig() throws IOException { } // rename tmpLogFile to mlog FileUtils.moveFile(tmpMLogFile, mlogFile); + + File oldMLogFile = SystemFileFactory.INSTANCE.getFile(SCHEMA_DIR + File.separator + + MetadataConstant.METADATA_OLD_LOG); + + if (oldMLogFile.delete()) { Review comment: true if and only if the file or directory is successfully deleted ```suggestion if (!oldMLogFile.delete()) { ``` ## File path: server/src/main/java/org/apache/iotdb/db/sync/receiver/transfer/SyncServiceImpl.java ## @@ -272,18 +274,25 @@ public SyncStatus checkDataMD5(String md5OfSender) throws TException { private void loadMetadata() { logger.info("Start to load metadata in sync process."); if (currentFile.get().exists()) { - try (BufferedReader br = new BufferedReader( - new java.io.FileReader(currentFile.get( { -String metadataOperation; -while ((metadataOperation = br.readLine()) != null) { + MLogReader mLogReader = null; + try { +mLogReader = new MLogReader(config.getSchemaDir(), MetadataConstant.METADATA_LOG); +while (mLogReader.hasNext()) { + PhysicalPlan plan = mLogReader.next(); try { -IoTDB.metaManager.operation(metadataOperation); - } catch (IOException | MetadataException e) { -logger.error("Can not operate metadata operation {} ", metadataOperation, e); +if (plan == null) { + continue; +} +IoTDB.metaManager.operation(plan); + } catch (Exception e) { +logger.error("Can not operate metadata operation {} for err:{}", plan.getOperatorType(), e); } } +mLogReader.close(); } catch (IOException e) { logger.error("Cannot read the file {}.", currentFile.get().getAbsoluteFile(), e); + } finally { +mLogReader.close(); Review comment: with `finally`, we could close `mLogReader` only once ## File path: server/src/main/java/org/apache/iotdb/db/metadata/logfile/MLogWriter.java ## @@ -0,0 +1,350 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one + * or more contributor license agreements. See the NOTICE file + * distributed with this work for additional information + * regarding copyright ownership. The ASF licenses this file + * to you under the Apache License, Version 2.0 (the + * "License"); you may not use this file except in compliance + * with the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, + * software distributed under the License is distributed on an + * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY + * KIND, either express or implied. See the License for the + * specific language governing permissions and limitations + * under the License. + */ +package org.apache.iotdb.db.metadata.logfile; + +import java.io.*; +import java.nio.BufferOverflowException; +import java.nio.ByteBuffer; +import java.nio.file.Files; +import java.util.*; + +import org.apache.iotdb.db.conf.IoTDBDescriptor; +import org.apache.iotdb.db.engine.fileSystem.SystemFileFactory; +import org.apache.iotdb.db.exception.metadata.MetadataException; +import org.apache.iotdb.db.metadata.MetadataConstant; +import org.apache.iotdb.db.metadata.MetadataOperationType; +import org.apache.iotdb.db.metadata.PartialPath; +import org.apache.iotdb.db.metadata.mnode.MNode; +import org.apache.iotdb.db.metadata.mnode.MeasurementMNode; +import org.apache.iotdb.db.metadata.mnode.StorageGroupMNode; +import org.apache.iotdb.db.qp.physical.PhysicalPlan; +import org.apache.iotdb.db.qp.physical.sys.*; +import org.apache.iotdb.db.writelog.io.LogWriter; +import org.apache.iotdb.tsfile.file.metadata.enums.CompressionType; +import org.apache.iotdb.tsfile.file.metadata.enums.TSDataType; +import org.apache.iotdb.tsfile.file.metadata.enums.TSEncoding; +import org.apache.iotdb.tsfile.fileSystem.FSFactoryProducer; +import org.slf4j.Logger; +import org.slf4j.LoggerFactory; + +public class MLogWriter { + + private static final Logger logger = LoggerFactory.getLogger(MLogWriter.class); + private File logFile; + private LogWriter logWriter; + private int logNum; + private ByteBuffer mlogBuffer = ByteBuffer.allocate( +IoTDBDescriptor.getInstance().getConfig().getMlogBufferSize()); + + public MLogWriter(String schemaDir, String logFileName) throws IOException { +File metadataDir = SystemFileFactory.INSTANCE.getFile(schemaDir); +if (!metadataDir.exists()) { + if (metadataDir.mkdirs()) { +
[GitHub] [incubator-iotdb] qiaojialin merged pull request #1720: [IOTDB-871] change port to rpc port in session
qiaojialin merged pull request #1720: URL: https://github.com/apache/incubator-iotdb/pull/1720 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] jt2594838 commented on a change in pull request #1698: [IoTDB-850] check list user/role privilege
jt2594838 commented on a change in pull request #1698: URL: https://github.com/apache/incubator-iotdb/pull/1698#discussion_r486717381 ## File path: server/src/main/java/org/apache/iotdb/db/qp/executor/PlanExecutor.java ## @@ -1116,14 +1125,26 @@ private ListDataSet executeListRole() { return dataSet; } - private ListDataSet executeListUser() { -List userList = authorizer.listAllUsers(); + private ListDataSet executeListUser(AuthorPlan plan) throws AuthException { +List paths = new ArrayList<>(); +paths.add(plan.getNodeName()); +// check if current user is granted list_user privilege Review comment: Better replaced with `Collections.singletonList()` ## File path: server/src/main/java/org/apache/iotdb/db/qp/executor/PlanExecutor.java ## @@ -1116,14 +1125,26 @@ private ListDataSet executeListRole() { return dataSet; } - private ListDataSet executeListUser() { -List userList = authorizer.listAllUsers(); + private ListDataSet executeListUser(AuthorPlan plan) throws AuthException { +List paths = new ArrayList<>(); +paths.add(plan.getNodeName()); +// check if current user is granted list_user privilege +boolean hasListUserPrivilege = AuthorityChecker +.check(plan.getLoginUserName(), paths, plan.getOperatorType(), plan.getLoginUserName()); + List headerList = new ArrayList<>(); List typeList = new ArrayList<>(); headerList.add(new PartialPath(COLUMN_USER, false)); typeList.add(TSDataType.TEXT); -int index = 0; ListDataSet dataSet = new ListDataSet(headerList, typeList); + +if (!hasListUserPrivilege) { + return dataSet; +} + +List userList = authorizer.listAllUsers(); +int index = 0; Review comment: What is this index for? ## File path: server/src/main/java/org/apache/iotdb/db/qp/physical/sys/AuthorPlan.java ## @@ -39,12 +39,13 @@ public class AuthorPlan extends PhysicalPlan { private AuthorOperator.AuthorType authorType; - private String userName; private String roleName; private String password; private String newPassword; private Set permissions; private PartialPath nodeName; + private String userName; + private String loginUserName; Review comment: I think maybe we can push loginUserName down to PhysicalPlan. It is often meaningful to know who issued this plan. ## File path: server/src/main/java/org/apache/iotdb/db/qp/executor/PlanExecutor.java ## @@ -1098,13 +1099,21 @@ protected QueryDataSet processAuthorQuery(AuthorPlan plan) return dataSet; } - private ListDataSet executeListRole() { + private ListDataSet executeListRole(AuthorPlan plan) throws AuthException { int index = 0; List headerList = new ArrayList<>(); List typeList = new ArrayList<>(); headerList.add(new PartialPath(COLUMN_ROLE, false)); typeList.add(TSDataType.TEXT); ListDataSet dataSet = new ListDataSet(headerList, typeList); + +boolean hasListRolePrivilege = AuthorityChecker +.check(plan.getLoginUserName(), new ArrayList<>(), plan.getOperatorType(), plan.getLoginUserName()); Review comment: Better replaced with `Collections.emptyList()`. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] haimeiguo commented on a change in pull request #1698: [IoTDB-850] check list user/role privilege
haimeiguo commented on a change in pull request #1698: URL: https://github.com/apache/incubator-iotdb/pull/1698#discussion_r486717423 ## File path: server/src/test/java/org/apache/iotdb/db/integration/IoTDBAuthorizationIT.java ## @@ -793,15 +793,19 @@ public void testListRolePrivileges() throws ClassNotFoundException, SQLException try { adminStmt.execute("CREATE ROLE role1"); - adminStmt.execute( + ResultSet resultSet = adminStmt.executeQuery("LIST ROLE PRIVILEGES role1"); + String ans = ""; + try { +//not granted list role privilege, should return empty +validateResultSet(resultSet, ans); + +adminStmt.execute( "GRANT ROLE role1 PRIVILEGES 'READ_TIMESERIES','INSERT_TIMESERIES','DELETE_TIMESERIES' ON root.a.b.c"); - adminStmt.execute( +adminStmt.execute( Review comment: done. Thank you :) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] removed a comment on pull request #460: [IOTDB-68] New shared-nothing cluster
sonarcloud[bot] removed a comment on pull request #460: URL: https://github.com/apache/incubator-iotdb/pull/460#issuecomment-690232861 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) [4 Security Hotspots](https://sonarcloud.io/project/issue s?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [84 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) [0.9% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] commented on pull request #460: [IOTDB-68] New shared-nothing cluster
sonarcloud[bot] commented on pull request #460: URL: https://github.com/apache/incubator-iotdb/pull/460#issuecomment-690264150 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) [4 Security Hotspots](https://sonarcloud.io/project/issue s?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [84 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) [0.9% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] removed a comment on pull request #460: [IOTDB-68] New shared-nothing cluster
sonarcloud[bot] removed a comment on pull request #460: URL: https://github.com/apache/incubator-iotdb/pull/460#issuecomment-690115956 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) [4 Security Hotspots](https://sonarcloud.io/project/issue s?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [84 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) [0.9% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] commented on pull request #460: [IOTDB-68] New shared-nothing cluster
sonarcloud[bot] commented on pull request #460: URL: https://github.com/apache/incubator-iotdb/pull/460#issuecomment-690232861 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) [4 Security Hotspots](https://sonarcloud.io/project/issue s?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [84 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) [0.9% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] qiaojialin merged pull request #1713: Python method "set_time_zone" exception message
qiaojialin merged pull request #1713: URL: https://github.com/apache/incubator-iotdb/pull/1713 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] qiaojialin merged pull request #1697: Fix As clause doc
qiaojialin merged pull request #1697: URL: https://github.com/apache/incubator-iotdb/pull/1697 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] qiaojialin merged pull request #1705: [IOTDB-873] Add count devices DDL
qiaojialin merged pull request #1705: URL: https://github.com/apache/incubator-iotdb/pull/1705 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] qiaojialin merged pull request #1712: Add merge limiting
qiaojialin merged pull request #1712: URL: https://github.com/apache/incubator-iotdb/pull/1712 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] haimeiguo commented on a change in pull request #1720: [iotdb-871] change port to rpc port in session
haimeiguo commented on a change in pull request #1720: URL: https://github.com/apache/incubator-iotdb/pull/1720#discussion_r486254431 ## File path: docs/zh/UserGuide/Client/Programming - TsFile API.md ## @@ -175,7 +175,7 @@ TsFile可以通过以下三个步骤生成,完整的代码参见"写入 TsFile ``` 在上面的例子中,数据文件将存储在 HDFS 中,而不是本地文件系统中。如果你想在本地文件系统中存储数据文件,你可以使用`conf.setTSFileStorageFs("LOCAL")`,这也是默认的配置。 -您还可以通过`config.setHdfsIp(...)`和`config.setHdfsPort(...)`来配置 HDFS 的 IP 和端口。默认的 IP是`localhost`,默认的端口是`9000`. +您还可以通过`config.setHdfsIp(...)`和`config.setHdfsPort(...)`来配置 HDFS 的 IP 和端口。默认的 IP是`localhost`,默认的rpc端口是`9000`. Review comment: done. Thank you:) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] jixuan1989 commented on issue #1719: IoTDB是否支持自动重连功能 (Does Session support reconnect?)
jixuan1989 commented on issue #1719: URL: https://github.com/apache/incubator-iotdb/issues/1719#issuecomment-690152455 If you want to reconnect the server if the network is broken because the network problem or some others in the client side, it is ok to use sessionPool in 0.10.1. But there is a bug for reconnecting the server (if the server is restarted) when using sessionPool in 0.10.1. you can compile source code from rel/0.10 or master branch to get a bug-fix version. 如果你想在客户端网络出问题时重连,0.10.1的sessionPool就可以。 但是这个版本在服务器重启的情况下,sessionPool有个重连的bug。已经在rel/0.10和master分支上修复了。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] commented on pull request #460: [IOTDB-68] New shared-nothing cluster
sonarcloud[bot] commented on pull request #460: URL: https://github.com/apache/incubator-iotdb/pull/460#issuecomment-690115956 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) [4 Security Hotspots](https://sonarcloud.io/project/issue s?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [84 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) [0.9% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] removed a comment on pull request #460: [IOTDB-68] New shared-nothing cluster
sonarcloud[bot] removed a comment on pull request #460: URL: https://github.com/apache/incubator-iotdb/pull/460#issuecomment-689981742 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) [4 Security Hotspots](https://sonarcloud.io/project/issue s?id=apache_incubator-iotdb=460=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [83 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=460=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) [1.1% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=460=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow opened a new pull request #1721: [IOTDB-868] fix comma bug for mlog
mychaow opened a new pull request #1721: URL: https://github.com/apache/incubator-iotdb/pull/1721 After we import the double-quoted path, users may have any characters in the path that includes ",". If we still record the mlog using csv format, we could not operate correctly to paths like root.changde.e34c49.struct@VALUE%PRESENT_ANGLE,_CUTTING_HEAD] The mlog needs to be encoded. https://issues.apache.org/jira/browse/IOTDB-868 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] LebronAl edited a comment on pull request #1691: [IOTDB-814] Cache and redirect distributed IoTDB's leader for write requests in IoTDB's client
LebronAl edited a comment on pull request #1691: URL: https://github.com/apache/incubator-iotdb/pull/1691#issuecomment-689985530 # Benchmark Parameter CLIENT_NUMBER=1 GROUP_NUMBER=1 DEVICE_NUMBER=20 SENSOR_NUMBER=20 BATCH_SIZE=50 LOOP=1000 DATA_TYPE=DOUBLE INSERT_MODE=session # Cluster config default_replica_num=1 enablePartition=false two nodes's cluster(34,35) # Cluster_new's latest Version(df8e0891cb75e0c6ff61ce10aedbaa8b99b66a31 add documents) ## forward(benchmark writes data to 34, sg belongs to 35,internal forward) 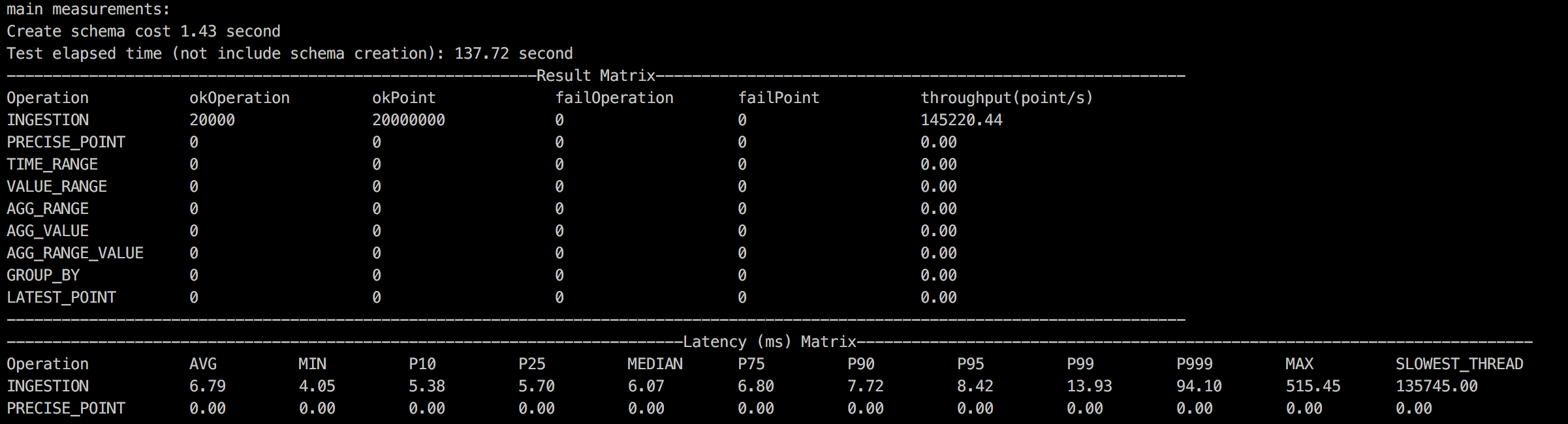 ## non-forward(benchmark writes data to 35, sg belongs to 35,no need to forward) 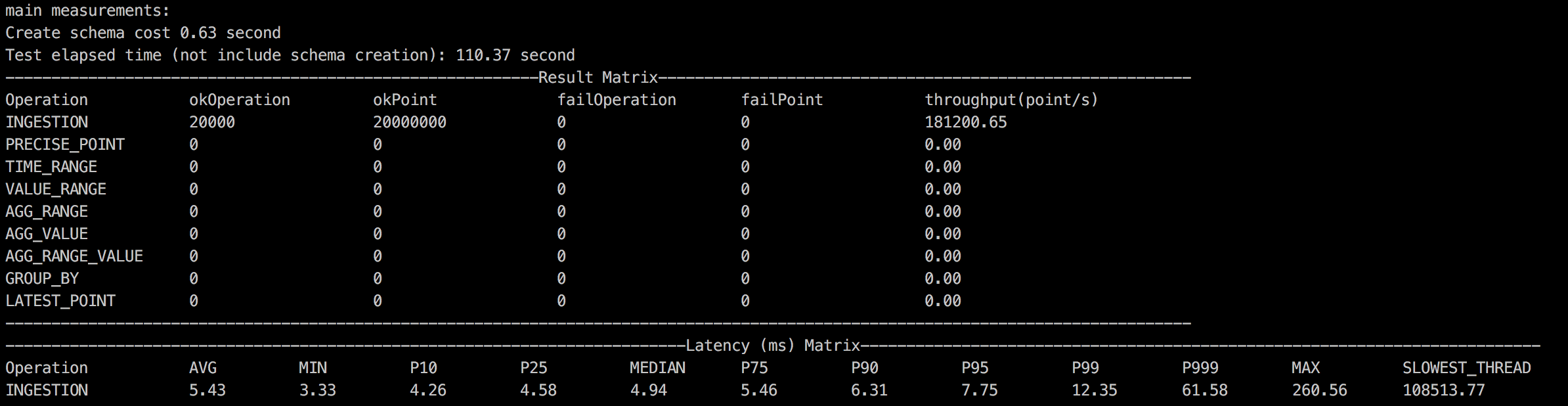 # Cluster_new_cache_leader's latest Version ## Close cache leader ### forward(benchmark writes data to 34, sg belongs to 35,internal forward) 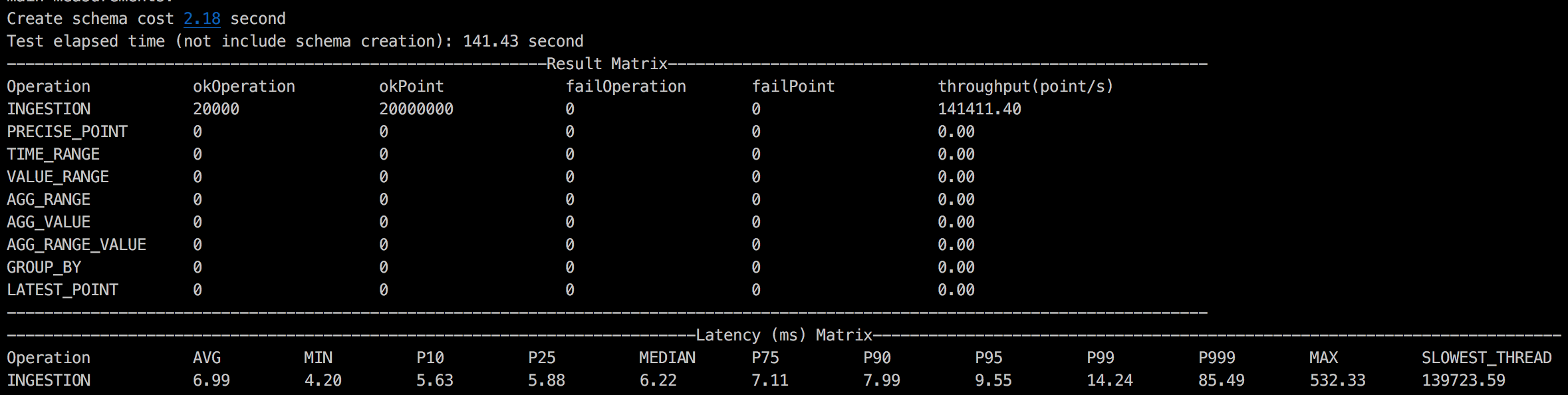 ### non-forward(benchmark writes data to 35, sg belongs to 35,no need to forward) 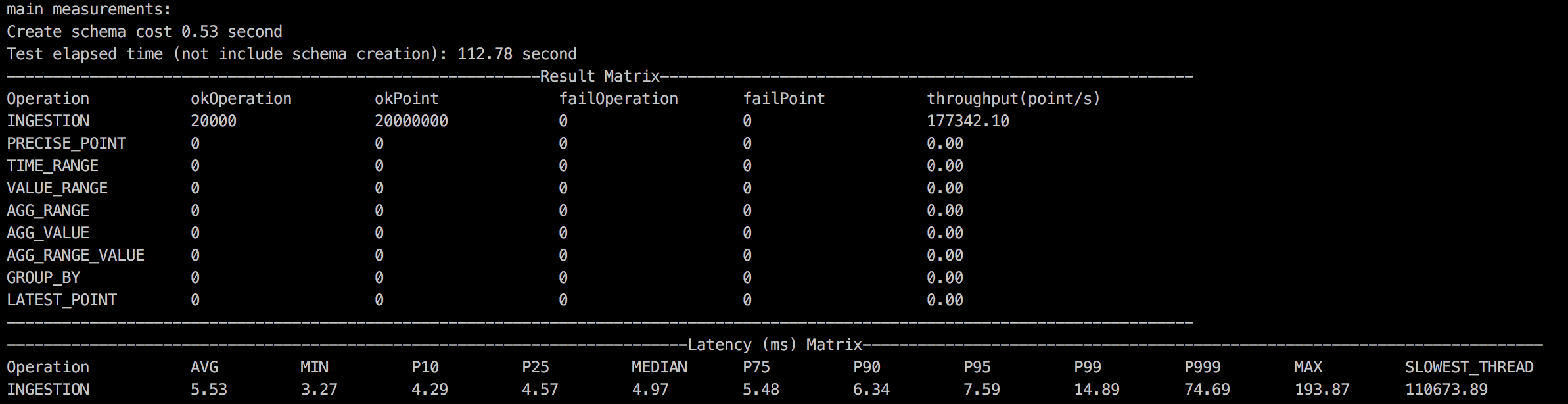 ## Open cache leader ### little forward(benchmark writes data to 34, sg belongs to 35,internal forward first write for each deviceId + cache leader) 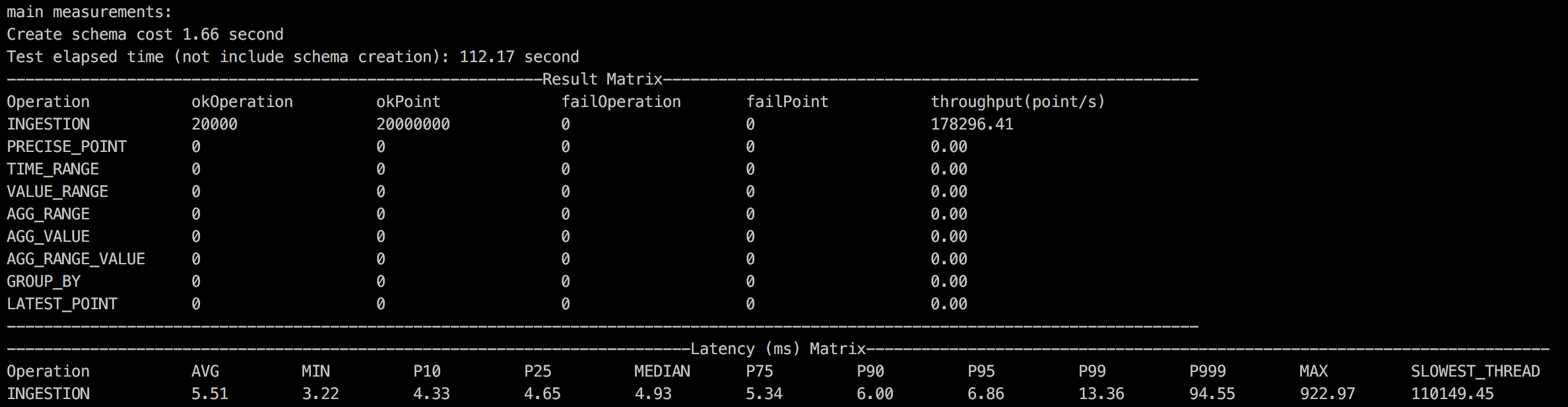 ### non-forward(benchmark writes data to 35, sg belongs to 35,no need to forward) 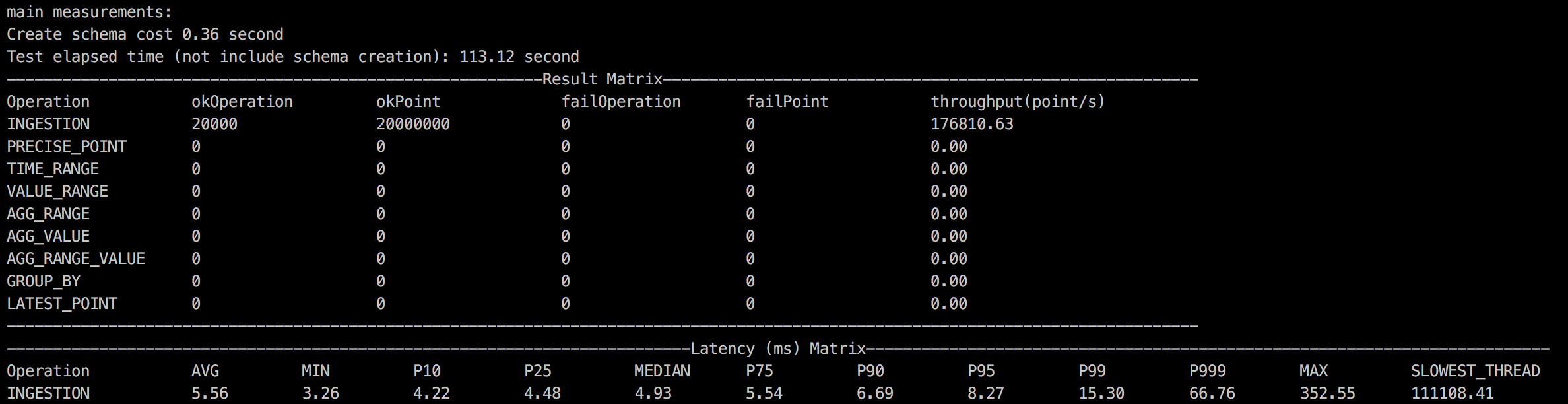 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] LebronAl commented on a change in pull request #1710: Supplement the document
LebronAl commented on a change in pull request #1710: URL: https://github.com/apache/incubator-iotdb/pull/1710#discussion_r486151502 ## File path: docs/zh/UserGuide/Operation Manual/DDL Data Definition Language.md ## @@ -41,14 +41,15 @@ Msg: org.apache.iotdb.exception.MetadataErrorException: org.apache.iotdb.excepti ## 查看存储组 -在存储组创建后,我们可以使用[SHOW STORAGE GROUP](../Operation%20Manual/SQL%20Reference.md)语句来查看所有的存储组,SQL语句如下所示: +在存储组创建后,我们可以使用[SHOW STORAGE GROUP](../Operation%20Manual/SQL%20Reference.md)语句和[SHOW STORAGE GROUP ](../Operation%20Manual/SQL%20Reference.md)来查看存储组,SQL语句如下所示: Review comment:  It seems that the `` doesn't display properly. ## File path: docs/UserGuide/Operation Manual/DDL Data Definition Language.md ## @@ -41,14 +41,15 @@ Msg: org.apache.iotdb.exception.MetadataErrorException: org.apache.iotdb.excepti ## Show Storage Group -After the storage group is created, we can use the [SHOW STORAGE GROUP](../Operation%20Manual/SQL%20Reference.md) statement to view all the storage groups. The SQL statement is as follows: +After the storage group is created, we can use the [SHOW STORAGE GROUP](../Operation%20Manual/SQL%20Reference.md) statement and [SHOW STORAGE GROUP ](../Operation%20Manual/SQL%20Reference.md) to view the storage groups. The SQL statement is as follows: Review comment: same as below ## File path: docs/UserGuide/Operation Manual/DDL Data Definition Language.md ## @@ -309,6 +310,20 @@ IoTDB> unset ttl to root.ln After unset TTL, all data will be accepted in `root.ln` +## Show TTL Review comment: same as neuyilan's suggestion~ This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] LebronAl commented on a change in pull request #1720: [iotdb-871] change port to rpc port in session
LebronAl commented on a change in pull request #1720: URL: https://github.com/apache/incubator-iotdb/pull/1720#discussion_r486141223 ## File path: docs/zh/UserGuide/Client/Programming - TsFile API.md ## @@ -175,7 +175,7 @@ TsFile可以通过以下三个步骤生成,完整的代码参见"写入 TsFile ``` 在上面的例子中,数据文件将存储在 HDFS 中,而不是本地文件系统中。如果你想在本地文件系统中存储数据文件,你可以使用`conf.setTSFileStorageFs("LOCAL")`,这也是默认的配置。 -您还可以通过`config.setHdfsIp(...)`和`config.setHdfsPort(...)`来配置 HDFS 的 IP 和端口。默认的 IP是`localhost`,默认的端口是`9000`. +您还可以通过`config.setHdfsIp(...)`和`config.setHdfsPort(...)`来配置 HDFS 的 IP 和端口。默认的 IP是`localhost`,默认的rpc端口是`9000`. Review comment: maybe `RPC` is better here~ This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] ATM006 commented on issue #1719: IoTDB是否支持自动重连功能 (Does Session support reconnect?)
ATM006 commented on issue #1719: URL: https://github.com/apache/incubator-iotdb/issues/1719#issuecomment-690063057 我用的是session而不是 SessionPool,我先试一下SessionPool。有问题再回来~ This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] qiaojialin commented on issue #1719: IoTDB是否支持自动重连功能 (Does Session support reconnect?)
qiaojialin commented on issue #1719: URL: https://github.com/apache/incubator-iotdb/issues/1719#issuecomment-690061364 只有 SessionPool 有自动重连,如果镜像有 SessionPool,那应该是支持的。自动断开的日志有吗 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] ATM006 commented on issue #1719: IoTDB是否支持自动重连功能 (Does Session support reconnect?)
ATM006 commented on issue #1719: URL: https://github.com/apache/incubator-iotdb/issues/1719#issuecomment-690059106 我用的是0.10.0版本的docker镜像session ,这个版本的镜像支持自动重连吗 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] mychaow commented on a change in pull request #1698: [IoTDB-850] check list user/role privilege
mychaow commented on a change in pull request #1698: URL: https://github.com/apache/incubator-iotdb/pull/1698#discussion_r486133953 ## File path: server/src/test/java/org/apache/iotdb/db/integration/IoTDBAuthorizationIT.java ## @@ -793,15 +793,19 @@ public void testListRolePrivileges() throws ClassNotFoundException, SQLException try { adminStmt.execute("CREATE ROLE role1"); - adminStmt.execute( + ResultSet resultSet = adminStmt.executeQuery("LIST ROLE PRIVILEGES role1"); + String ans = ""; + try { +//not granted list role privilege, should return empty +validateResultSet(resultSet, ans); + +adminStmt.execute( "GRANT ROLE role1 PRIVILEGES 'READ_TIMESERIES','INSERT_TIMESERIES','DELETE_TIMESERIES' ON root.a.b.c"); - adminStmt.execute( +adminStmt.execute( Review comment: delete the blank space. ## File path: server/src/main/java/org/apache/iotdb/db/qp/executor/PlanExecutor.java ## @@ -1059,7 +1060,7 @@ protected boolean deleteStorageGroups(DeleteStorageGroupPlan deleteStorageGroupP } return true; } - + Review comment: delete the blank space. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] qiaojialin commented on issue #1719: IoTDB是否支持自动重连功能
qiaojialin commented on issue #1719: URL: https://github.com/apache/incubator-iotdb/issues/1719#issuecomment-690054475 SessionPool 支持重连,不过如果客户端爆了运行异常,会连接泄漏,可以拉一下 rel/0.10 分支手动打包,在0.10.2-SNAPSHOT 版本修了 The SessionPool could reconnect when meet error. However, if you have RunTimeException in client, the connection will leak, we fixed this in rel/0.10 (0.10.2-SNAPSHOT). 另外,能提供复现程序吗? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] haimeiguo opened a new pull request #1720: [iotdb-870] change port to rpc port in session
haimeiguo opened a new pull request #1720: URL: https://github.com/apache/incubator-iotdb/pull/1720 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] ATM006 commented on issue #1719: IoTDB是否支持自动重连功能
ATM006 commented on issue #1719: URL: https://github.com/apache/incubator-iotdb/issues/1719#issuecomment-690041768 500qps出现session自动断开 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] commented on pull request #1650: [IOTDB-848] support order by time asc/desc
sonarcloud[bot] commented on pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#issuecomment-690034269 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [2 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) [0.0% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] removed a comment on pull request #1650: [IOTDB-848] support order by time asc/desc
sonarcloud[bot] removed a comment on pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#issuecomment-690018794 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [3 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) [0.0% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] commented on pull request #1650: [IOTDB-848] support order by time asc/desc
sonarcloud[bot] commented on pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#issuecomment-690018794 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [3 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) [0.0% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] removed a comment on pull request #1650: [IOTDB-848] support order by time asc/desc
sonarcloud[bot] removed a comment on pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#issuecomment-690010690 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [5 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) [0.0% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] LebronAl edited a comment on pull request #1691: [IOTDB-814] Cache and redirect distributed IoTDB's leader for write requests in IoTDB's client
LebronAl edited a comment on pull request #1691: URL: https://github.com/apache/incubator-iotdb/pull/1691#issuecomment-690013673 @jt2594838 @neuyilan @Ring-k @qiaojialin PTAL This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] LebronAl commented on pull request #1691: [IOTDB-814] Cache and redirect distributed IoTDB's leader for write requests in IoTDB's client
LebronAl commented on pull request #1691: URL: https://github.com/apache/incubator-iotdb/pull/1691#issuecomment-690013673 @jt2594838 @neuyilan @kr11 @qiaojialin PTAL This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] LebronAl edited a comment on pull request #1713: Python method "set_time_zone" exception message
LebronAl edited a comment on pull request #1713: URL: https://github.com/apache/incubator-iotdb/pull/1713#issuecomment-689913410 [Python sessionUT](https://github.com/apache/incubator-iotdb/pull/1578) is still under reviewing. You can take a review~ Thanks~ This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] commented on pull request #1650: [IOTDB-848] support order by time asc/desc
sonarcloud[bot] commented on pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#issuecomment-690010690 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [5 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) [0.0% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] sonarcloud[bot] removed a comment on pull request #1650: [IOTDB-848] support order by time asc/desc
sonarcloud[bot] removed a comment on pull request #1650: URL: https://github.com/apache/incubator-iotdb/pull/1650#issuecomment-689958738 Kudos, SonarCloud Quality Gate passed! [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [0 Bugs](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=BUG) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) [0 Vulnerabilities](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=VULNERABILITY) (and [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) [0 Security Hotspots](https://sonarcloud.io/project/i ssues?id=apache_incubator-iotdb=1650=false=SECURITY_HOTSPOT) to review) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [20 Code Smells](https://sonarcloud.io/project/issues?id=apache_incubator-iotdb=1650=false=CODE_SMELL) [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650) No Coverage information [](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) [0.0% Duplication](https://sonarcloud.io/component_measures?id=apache_incubator-iotdb=1650=new_duplicated_lines_density=list) The version of Java (1.8.0_252) you have used to run this analysis is deprecated and we will stop accepting it from October 2020. Please update to at least Java 11. Read more [here](https://sonarcloud.io/documentation/upcoming/) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [incubator-iotdb] liutaohua commented on issue #1640: IoTDB是否支持ORDER排序功能
liutaohua commented on issue #1640: URL: https://github.com/apache/incubator-iotdb/issues/1640#issuecomment-690009628 > 请问当前0.10.1版本支持了吗 不支持,目前还没有把pr合并到master This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org