[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r839413410 ## File path: flink-runtime/src/test/java/org/apache/flink/runtime/metrics/filter/DefaultMetricFilterTest.java ## @@ -0,0 +1,192 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + *http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package org.apache.flink.runtime.metrics.filter; + +import org.apache.flink.configuration.Configuration; +import org.apache.flink.configuration.MetricOptions; +import org.apache.flink.metrics.Counter; +import org.apache.flink.metrics.Meter; +import org.apache.flink.metrics.MetricType; +import org.apache.flink.metrics.util.TestCounter; +import org.apache.flink.metrics.util.TestMeter; + +import org.junit.jupiter.api.Test; +import org.junit.jupiter.api.parallel.Execution; +import org.junit.jupiter.api.parallel.ExecutionMode; + +import java.util.Arrays; +import java.util.EnumSet; +import java.util.regex.Pattern; + +import static org.assertj.core.api.Assertions.assertThat; + +@Execution(ExecutionMode.CONCURRENT) +class DefaultMetricFilterTest { + +private static final Counter COUNTER = new TestCounter(); +private static final Meter METER = new TestMeter(); + +@Test +void testConvertToPatternWithoutWildcards() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecordsIn"); +assertThat(pattern.toString()).isEqualTo("(numRecordsIn)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(false); +} + +@Test +void testConvertToPatternSingle() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecords*"); +assertThat(pattern.toString()).isEqualTo("(numRecords.+)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(false); +} + +@Test +void testConvertToPatternMultiple() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecords*;numBytes*"); +assertThat(pattern.toString()).isEqualTo("(numRecords.+|numBytes.+)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytes").matches()).isEqualTo(false); +assertThat(pattern.matcher("hello").matches()).isEqualTo(false); +} + +@Test +void testParseMetricTypesSingle() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter"); +assertThat(types).containsExactly(MetricType.METER); +} + +@Test +void testParseMetricTypesMultiple() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter;counter"); +assertThat(types).containsExactlyInAnyOrder(MetricType.METER, MetricType.COUNTER); +} + +@Test +void testParseMetricTypesCaseIgnored() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter;CoUnTeR"); +assertThat(types).containsExactlyInAnyOrder(MetricType.METER, MetricType.COUNTER); +} + +@Test +void testFromConfigurationIncludeByScope() { +Configuration configuration = new Configuration(); +configuration.set( +MetricOptions.REPORTER_INCLUDES, Arrays.asList("include1:*:*", "include2.*:*:*")); + +final MetricFilter metricFilter = DefaultMetricFilter.fromConfiguration(configuration); + +assertThat(metricFilter.filter(COUNTER, "name", "include1")).isEqualTo(true); +assertThat(metricFilter.filter(COUNTER, "name", "include1.bar")).isEqualTo(false); +assertThat(metricFilter.filter(COUNTER, "name", "include2")).isEqualTo(false); +assertThat(metricFilter.filter(COUNTER, "name", "include2.bar")).isEqualTo(true); +} + +@Test +void testFromConfigurationIncludeByName() { +Configuration configuration = new Configuration(); +configuration.set(MetricOptions.REPORTER_INCLUDES, Arrays.asList("*:name:*")); + +final MetricFilter metricFilter =
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r839393270 ## File path: flink-core/src/main/java/org/apache/flink/configuration/MetricOptions.java ## @@ -117,8 +119,88 @@ .withDescription( "The set of variables that should be excluded for the reporter named . Only applicable to tag-based reporters (e.g., PRometheus, InfluxDB)."); +private static final Description.DescriptionBuilder addFilterDescription( +Description.DescriptionBuilder description) { +return description +.text("Filters are specified as a list, with each filter following this format:") +.linebreak() +.text("%s", code("[:[;][:type[;type#N]]]")) +.list( +text( +"scope: Filters based on the logical scope.%s" ++ "Specified as a pattern where %s matches one or more characters and %s separates scope components.%s" Review comment: Without a line break it becomes too much of a mess imo. 2 line breaks worked better, but looked a bit oodd because there now was an empty line within the paragraph, but none at the end (i.e., between list items). I now added 2 more linebreaks to the end of each list item: 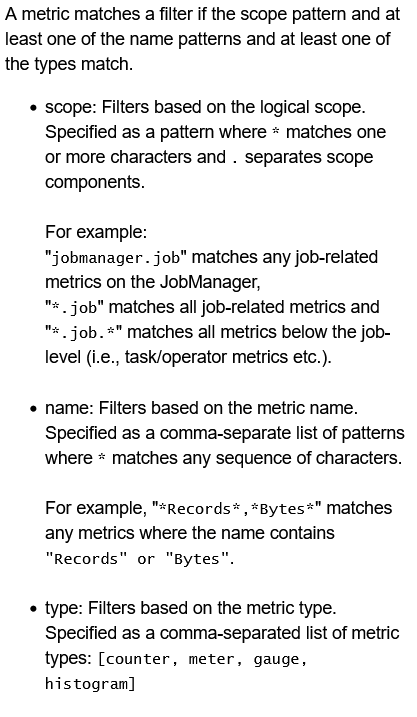 -- This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. To unsubscribe, e-mail: issues-unsubscr...@flink.apache.org For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r839398527 ## File path: flink-runtime/src/main/java/org/apache/flink/runtime/metrics/filter/DefaultMetricFilter.java ## @@ -0,0 +1,131 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + *http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package org.apache.flink.runtime.metrics.filter; + +import org.apache.flink.annotation.VisibleForTesting; +import org.apache.flink.configuration.Configuration; +import org.apache.flink.configuration.MetricOptions; +import org.apache.flink.metrics.Metric; +import org.apache.flink.metrics.MetricType; + +import java.util.Arrays; +import java.util.EnumSet; +import java.util.List; +import java.util.Locale; +import java.util.regex.Pattern; +import java.util.stream.Collectors; + +/** + * Default {@link MetricFilter} implementation that filters metrics based on {@link + * MetricOptions#REPORTER_INCLUDES}/{@link MetricOptions#REPORTER_EXCLUDES}. + */ +public class DefaultMetricFilter implements MetricFilter { + +private static final EnumSet ALL_METRIC_TYPES = EnumSet.allOf(MetricType.class); +private static final EnumSet NO_METRIC_TYPES = EnumSet.noneOf(MetricType.class); + +private final List includes; +private final List excludes; + +private DefaultMetricFilter(List includes, List excludes) { +this.includes = includes; +this.excludes = excludes; +} + +@Override +public boolean filter(Metric metric, String name, String logicalScope) { +for (FilterSpec exclude : excludes) { +if (exclude.namePattern.matcher(name).matches() +&& exclude.scopePattern.matcher(logicalScope).matches() +&& exclude.types.contains(metric.getMetricType())) { +return false; +} +} +for (FilterSpec include : includes) { +if (include.namePattern.matcher(name).matches() +&& include.scopePattern.matcher(logicalScope).matches() +&& include.types.contains(metric.getMetricType())) { +return true; +} +} +return false; +} + +public static MetricFilter fromConfiguration(Configuration configuration) { +final List includes = configuration.get(MetricOptions.REPORTER_INCLUDES); +final List excludes = configuration.get(MetricOptions.REPORTER_EXCLUDES); + +final List includeFilters = +includes.stream().map(i -> parse(i)).collect(Collectors.toList()); +final List excludeFilters = +excludes.stream().map(e -> parse(e)).collect(Collectors.toList()); + +return new DefaultMetricFilter(includeFilters, excludeFilters); +} + +private static FilterSpec parse(String filter) { +final String[] split = filter.split(":"); +final Pattern scope = convertToPattern(split[0]); +final Pattern name = split.length > 1 ? convertToPattern(split[1]) : Pattern.compile(".+"); +final EnumSet type = +split.length > 2 ? parseMetricTypes(split[2]) : ALL_METRIC_TYPES; + +return new FilterSpec(scope, name, type); +} + +@VisibleForTesting +static Pattern convertToPattern(String scopeOrNameComponent) { +final String[] split = scopeOrNameComponent.split(";"); + +final String rawPattern = +Arrays.stream(split) +.map(s -> s.replaceAll("\\.", "\\.")) +.map(s -> s.replaceAll("\\*", ".+")) Review comment: It's not common, no. It isn't intuitive. I remember that I did intentionally switched to `+`, but that may have been due to an earlier iteration. I can't think of a reason to not use `*` now. ## File path: flink-runtime/src/main/java/org/apache/flink/runtime/metrics/filter/DefaultMetricFilter.java ## @@ -0,0 +1,131 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + *
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r839393270 ## File path: flink-core/src/main/java/org/apache/flink/configuration/MetricOptions.java ## @@ -117,8 +119,88 @@ .withDescription( "The set of variables that should be excluded for the reporter named . Only applicable to tag-based reporters (e.g., PRometheus, InfluxDB)."); +private static final Description.DescriptionBuilder addFilterDescription( +Description.DescriptionBuilder description) { +return description +.text("Filters are specified as a list, with each filter following this format:") +.linebreak() +.text("%s", code("[:[;][:type[;type#N]]]")) +.list( +text( +"scope: Filters based on the logical scope.%s" ++ "Specified as a pattern where %s matches one or more characters and %s separates scope components.%s" Review comment: Without a line break it becomes too much of a mess imo. 2 line breaks worked better, but looked a bit because there now was an empty line within the paragraph, but none at the end (i.e., between list items). I now added 2 more linebreaks to the end of each list item: 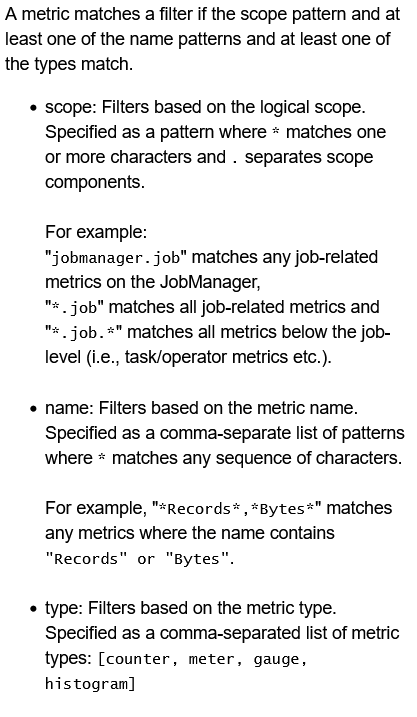 -- This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. To unsubscribe, e-mail: issues-unsubscr...@flink.apache.org For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r839376654 ## File path: flink-runtime/src/main/java/org/apache/flink/runtime/metrics/MetricRegistryImpl.java ## @@ -380,6 +382,12 @@ public void register(Metric metric, String metricName, AbstractMetricGroup group LOG.warn( "Cannot register metric, because the MetricRegistry has already been shut down."); } else { +if (LOG.isDebugEnabled()) { +LOG.debug( +"Registering metric {}.{}.", + group.getLogicalScope(CharacterFilter.NO_OP_FILTER), +metricName); +} Review comment: That's more or less a convention of the metric system where we (for better or worse) try to reduce the impact if the system isn't used at all. -- This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. To unsubscribe, e-mail: issues-unsubscr...@flink.apache.org For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r839272782 ## File path: flink-core/src/main/java/org/apache/flink/configuration/MetricOptions.java ## @@ -117,8 +119,88 @@ .withDescription( "The set of variables that should be excluded for the reporter named . Only applicable to tag-based reporters (e.g., PRometheus, InfluxDB)."); +private static final Description.DescriptionBuilder addFilterDescription( +Description.DescriptionBuilder description) { +return description +.text("Filters are specified as a list, with each filter following this format:") +.linebreak() +.text("%s", code("[:[;][:type[;type#N]]]")) Review comment: I should add a test for the parsing of multiple patterns; I'm afraid the nested `;` might trow of the parser. -- This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. To unsubscribe, e-mail: issues-unsubscr...@flink.apache.org For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r838814545 ## File path: flink-runtime/src/test/java/org/apache/flink/runtime/metrics/filter/DefaultMetricFilterTest.java ## @@ -0,0 +1,192 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + *http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package org.apache.flink.runtime.metrics.filter; + +import org.apache.flink.configuration.Configuration; +import org.apache.flink.configuration.MetricOptions; +import org.apache.flink.metrics.Counter; +import org.apache.flink.metrics.Meter; +import org.apache.flink.metrics.MetricType; +import org.apache.flink.metrics.util.TestCounter; +import org.apache.flink.metrics.util.TestMeter; + +import org.junit.jupiter.api.Test; +import org.junit.jupiter.api.parallel.Execution; +import org.junit.jupiter.api.parallel.ExecutionMode; + +import java.util.Arrays; +import java.util.EnumSet; +import java.util.regex.Pattern; + +import static org.assertj.core.api.Assertions.assertThat; + +@Execution(ExecutionMode.CONCURRENT) +class DefaultMetricFilterTest { + +private static final Counter COUNTER = new TestCounter(); +private static final Meter METER = new TestMeter(); + +@Test +void testConvertToPatternWithoutWildcards() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecordsIn"); +assertThat(pattern.toString()).isEqualTo("(numRecordsIn)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(false); +} + +@Test +void testConvertToPatternSingle() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecords*"); +assertThat(pattern.toString()).isEqualTo("(numRecords.+)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(false); +} + +@Test +void testConvertToPatternMultiple() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecords*;numBytes*"); +assertThat(pattern.toString()).isEqualTo("(numRecords.+|numBytes.+)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytes").matches()).isEqualTo(false); +assertThat(pattern.matcher("hello").matches()).isEqualTo(false); +} + +@Test +void testParseMetricTypesSingle() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter"); +assertThat(types).containsExactly(MetricType.METER); +} + +@Test +void testParseMetricTypesMultiple() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter;counter"); +assertThat(types).containsExactlyInAnyOrder(MetricType.METER, MetricType.COUNTER); +} + +@Test +void testParseMetricTypesCaseIgnored() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter;CoUnTeR"); +assertThat(types).containsExactlyInAnyOrder(MetricType.METER, MetricType.COUNTER); +} + +@Test +void testFromConfigurationIncludeByScope() { +Configuration configuration = new Configuration(); +configuration.set( +MetricOptions.REPORTER_INCLUDES, Arrays.asList("include1:*:*", "include2.*:*:*")); + +final MetricFilter metricFilter = DefaultMetricFilter.fromConfiguration(configuration); + +assertThat(metricFilter.filter(COUNTER, "name", "include1")).isEqualTo(true); +assertThat(metricFilter.filter(COUNTER, "name", "include1.bar")).isEqualTo(false); +assertThat(metricFilter.filter(COUNTER, "name", "include2")).isEqualTo(false); +assertThat(metricFilter.filter(COUNTER, "name", "include2.bar")).isEqualTo(true); +} + +@Test +void testFromConfigurationIncludeByName() { +Configuration configuration = new Configuration(); +configuration.set(MetricOptions.REPORTER_INCLUDES, Arrays.asList("*:name:*")); + +final MetricFilter metricFilter =
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r838813652 ## File path: flink-runtime/src/test/java/org/apache/flink/runtime/metrics/filter/DefaultMetricFilterTest.java ## @@ -0,0 +1,192 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + *http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package org.apache.flink.runtime.metrics.filter; + +import org.apache.flink.configuration.Configuration; +import org.apache.flink.configuration.MetricOptions; +import org.apache.flink.metrics.Counter; +import org.apache.flink.metrics.Meter; +import org.apache.flink.metrics.MetricType; +import org.apache.flink.metrics.util.TestCounter; +import org.apache.flink.metrics.util.TestMeter; + +import org.junit.jupiter.api.Test; +import org.junit.jupiter.api.parallel.Execution; +import org.junit.jupiter.api.parallel.ExecutionMode; + +import java.util.Arrays; +import java.util.EnumSet; +import java.util.regex.Pattern; + +import static org.assertj.core.api.Assertions.assertThat; + +@Execution(ExecutionMode.CONCURRENT) +class DefaultMetricFilterTest { + +private static final Counter COUNTER = new TestCounter(); +private static final Meter METER = new TestMeter(); + +@Test +void testConvertToPatternWithoutWildcards() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecordsIn"); +assertThat(pattern.toString()).isEqualTo("(numRecordsIn)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(false); +} + +@Test +void testConvertToPatternSingle() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecords*"); +assertThat(pattern.toString()).isEqualTo("(numRecords.+)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(false); +} + +@Test +void testConvertToPatternMultiple() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecords*;numBytes*"); +assertThat(pattern.toString()).isEqualTo("(numRecords.+|numBytes.+)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytes").matches()).isEqualTo(false); +assertThat(pattern.matcher("hello").matches()).isEqualTo(false); +} + +@Test +void testParseMetricTypesSingle() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter"); +assertThat(types).containsExactly(MetricType.METER); +} + +@Test +void testParseMetricTypesMultiple() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter;counter"); +assertThat(types).containsExactlyInAnyOrder(MetricType.METER, MetricType.COUNTER); +} + +@Test +void testParseMetricTypesCaseIgnored() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter;CoUnTeR"); +assertThat(types).containsExactlyInAnyOrder(MetricType.METER, MetricType.COUNTER); +} + +@Test +void testFromConfigurationIncludeByScope() { +Configuration configuration = new Configuration(); +configuration.set( +MetricOptions.REPORTER_INCLUDES, Arrays.asList("include1:*:*", "include2.*:*:*")); + +final MetricFilter metricFilter = DefaultMetricFilter.fromConfiguration(configuration); + +assertThat(metricFilter.filter(COUNTER, "name", "include1")).isEqualTo(true); +assertThat(metricFilter.filter(COUNTER, "name", "include1.bar")).isEqualTo(false); +assertThat(metricFilter.filter(COUNTER, "name", "include2")).isEqualTo(false); +assertThat(metricFilter.filter(COUNTER, "name", "include2.bar")).isEqualTo(true); +} + +@Test +void testFromConfigurationIncludeByName() { +Configuration configuration = new Configuration(); +configuration.set(MetricOptions.REPORTER_INCLUDES, Arrays.asList("*:name:*")); + +final MetricFilter metricFilter =
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r838812659 ## File path: flink-runtime/src/test/java/org/apache/flink/runtime/metrics/filter/DefaultMetricFilterTest.java ## @@ -0,0 +1,192 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + *http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package org.apache.flink.runtime.metrics.filter; + +import org.apache.flink.configuration.Configuration; +import org.apache.flink.configuration.MetricOptions; +import org.apache.flink.metrics.Counter; +import org.apache.flink.metrics.Meter; +import org.apache.flink.metrics.MetricType; +import org.apache.flink.metrics.util.TestCounter; +import org.apache.flink.metrics.util.TestMeter; + +import org.junit.jupiter.api.Test; +import org.junit.jupiter.api.parallel.Execution; +import org.junit.jupiter.api.parallel.ExecutionMode; + +import java.util.Arrays; +import java.util.EnumSet; +import java.util.regex.Pattern; + +import static org.assertj.core.api.Assertions.assertThat; + +@Execution(ExecutionMode.CONCURRENT) +class DefaultMetricFilterTest { + +private static final Counter COUNTER = new TestCounter(); +private static final Meter METER = new TestMeter(); + +@Test +void testConvertToPatternWithoutWildcards() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecordsIn"); +assertThat(pattern.toString()).isEqualTo("(numRecordsIn)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(false); +} + +@Test +void testConvertToPatternSingle() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecords*"); +assertThat(pattern.toString()).isEqualTo("(numRecords.+)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(false); +} + +@Test +void testConvertToPatternMultiple() { +final Pattern pattern = DefaultMetricFilter.convertToPattern("numRecords*;numBytes*"); +assertThat(pattern.toString()).isEqualTo("(numRecords.+|numBytes.+)"); +assertThat(pattern.matcher("numRecordsIn").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytesOut").matches()).isEqualTo(true); +assertThat(pattern.matcher("numBytes").matches()).isEqualTo(false); +assertThat(pattern.matcher("hello").matches()).isEqualTo(false); +} + +@Test +void testParseMetricTypesSingle() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter"); +assertThat(types).containsExactly(MetricType.METER); +} + +@Test +void testParseMetricTypesMultiple() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter;counter"); +assertThat(types).containsExactlyInAnyOrder(MetricType.METER, MetricType.COUNTER); +} + +@Test +void testParseMetricTypesCaseIgnored() { +final EnumSet types = DefaultMetricFilter.parseMetricTypes("meter;CoUnTeR"); +assertThat(types).containsExactlyInAnyOrder(MetricType.METER, MetricType.COUNTER); +} + +@Test +void testFromConfigurationIncludeByScope() { +Configuration configuration = new Configuration(); +configuration.set( +MetricOptions.REPORTER_INCLUDES, Arrays.asList("include1:*:*", "include2.*:*:*")); + +final MetricFilter metricFilter = DefaultMetricFilter.fromConfiguration(configuration); + +assertThat(metricFilter.filter(COUNTER, "name", "include1")).isEqualTo(true); +assertThat(metricFilter.filter(COUNTER, "name", "include1.bar")).isEqualTo(false); +assertThat(metricFilter.filter(COUNTER, "name", "include2")).isEqualTo(false); +assertThat(metricFilter.filter(COUNTER, "name", "include2.bar")).isEqualTo(true); +} + +@Test +void testFromConfigurationIncludeByName() { +Configuration configuration = new Configuration(); +configuration.set(MetricOptions.REPORTER_INCLUDES, Arrays.asList("*:name:*")); + +final MetricFilter metricFilter =
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r838807310 ## File path: flink-runtime/src/main/java/org/apache/flink/runtime/metrics/MetricRegistryImpl.java ## @@ -380,6 +382,12 @@ public void register(Metric metric, String metricName, AbstractMetricGroup group LOG.warn( "Cannot register metric, because the MetricRegistry has already been shut down."); } else { +if (LOG.isDebugEnabled()) { +LOG.debug( +"Registering metric {}.{}.", + group.getLogicalScope(CharacterFilter.NO_OP_FILTER), +metricName); +} Review comment: it is relatively expensive and permanently increases the memory footprint. -- This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. To unsubscribe, e-mail: issues-unsubscr...@flink.apache.org For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r838805255 ## File path: flink-core/src/main/java/org/apache/flink/configuration/MetricOptions.java ## @@ -117,8 +119,88 @@ .withDescription( "The set of variables that should be excluded for the reporter named . Only applicable to tag-based reporters (e.g., PRometheus, InfluxDB)."); +private static final Description.DescriptionBuilder addFilterDescription( +Description.DescriptionBuilder description) { +return description +.text("Filters are specified as a list, with each filter following this format:") +.linebreak() +.text("%s", code("[:[;][:type[;type#N]]]")) Review comment: It's up to the user so I don't see the harm. Personally I don't why `"*.job.task.operator:numRecords*;debloatedBufferSize;mailboxLatency*:meter;gauge"` is in any way less maintainable then `"*.job.task.operator:numRecords*:meter;gauge";"*.job.task.operator:debloatedBufferSize:meter;gauge";"*.job.task.operator:mailboxLatency*:meter;gauge"` Additionally, if we ever add support for proper yaml parsing then the ideal solution would likely be the following, which is closer to the current "powerful" option. ``` - filter: scope: *.job.task.operator:numRecords* names: - numRecords* - debloatedBufferSize - mailboxLatency* types: - meter - gauge ``` -- This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. To unsubscribe, e-mail: issues-unsubscr...@flink.apache.org For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r838805255 ## File path: flink-core/src/main/java/org/apache/flink/configuration/MetricOptions.java ## @@ -117,8 +119,88 @@ .withDescription( "The set of variables that should be excluded for the reporter named . Only applicable to tag-based reporters (e.g., PRometheus, InfluxDB)."); +private static final Description.DescriptionBuilder addFilterDescription( +Description.DescriptionBuilder description) { +return description +.text("Filters are specified as a list, with each filter following this format:") +.linebreak() +.text("%s", code("[:[;][:type[;type#N]]]")) Review comment: It's up to the user so I don't see the harm. Personally I don't why `"*.job.task.operator:numRecords*;debloatedBufferSize;mailboxLatency*:meter;gauge"` is in any way less maintainable then `"*.job.task.operator:numRecords*:meter;gauge;*.job.task.operator:debloatedBufferSize:meter;gauge;*.job.task.operator:mailboxLatency*:meter;gauge"` Additionally, if we ever add support for proper yaml parsing then the ideal solution would likely be the following, which is closer to the current "powerful" option. ``` - filter: scope: *.job.task.operator:numRecords* names: - numRecords* - debloatedBufferSize - mailboxLatency* types: - meter - gauge ``` -- This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. To unsubscribe, e-mail: issues-unsubscr...@flink.apache.org For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [flink] zentol commented on a change in pull request #19263: [FLINK-21585][metrics] Add options for in-/excluding metrics
zentol commented on a change in pull request #19263: URL: https://github.com/apache/flink/pull/19263#discussion_r838805255 ## File path: flink-core/src/main/java/org/apache/flink/configuration/MetricOptions.java ## @@ -117,8 +119,88 @@ .withDescription( "The set of variables that should be excluded for the reporter named . Only applicable to tag-based reporters (e.g., PRometheus, InfluxDB)."); +private static final Description.DescriptionBuilder addFilterDescription( +Description.DescriptionBuilder description) { +return description +.text("Filters are specified as a list, with each filter following this format:") +.linebreak() +.text("%s", code("[:[;][:type[;type#N]]]")) Review comment: It's up to the user so I don't see the harm. Personally I don't why `"*.job.task.operator:numRecords*;debloatedBufferSize;mailboxLatency*:meter;gauge` is in any way less maintainable then `"*.job.task.operator:numRecords*:meter;gauge;"*.job.task.operator:debloatedBufferSize:meter;gauge;"*.job.task.operator:mailboxLatency*:meter;gauge` Additionally, if we ever add support for proper yaml parsing then the ideal solution would likely be the following, which is closer to the current "powerful" option. ``` - filter: scope: *.job.task.operator:numRecords* names: - numRecords* - debloatedBufferSize - mailboxLatency* types: - meter - gauge ``` -- This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. To unsubscribe, e-mail: issues-unsubscr...@flink.apache.org For queries about this service, please contact Infrastructure at: us...@infra.apache.org